html下拉列表取值subject给后台python,后台python用一个fastapi的post接口接收,python代码中有许多get类型接口,这些get类型接口调用定义的函数,而这些函数需要用到html传的subject值,如何撰写代码?
时间: 2024-02-09 12:12:47 浏览: 23
可以按照以下步骤实现将 HTML 下拉列表的值传递给后台 Python,并在 Python 中调用相应的函数:
1. 在 HTML 中创建一个下拉列表,并添加一个提交按钮:
```
<form id="myForm">
<select id="dropdown" name="subject">
<option value="math">Math</option>
<option value="science">Science</option>
<option value="history">History</option>
</select>
<button type="submit">Submit</button>
</form>
```
2. 在 JavaScript 中获取下拉列表的值,并使用 AJAX 将数据提交到后台 Python。例如:
```
var form = document.getElementById("myForm");
form.addEventListener("submit", function(event) {
event.preventDefault(); // prevent the form from submitting normally
var selectedSubject = document.getElementById("dropdown").value;
$.ajax({
type: "POST",
url: "/submit",
data: { subject: selectedSubject },
success: function(response) {
console.log(response);
}
});
});
```
3. 在 Python 中使用 FastAPI 创建一个 POST 接口,并定义相应的函数。例如:
```
from fastapi import FastAPI, Form
app = FastAPI()
@app.post("/submit")
async def submit_form(subject: str = Form(...)):
if subject == "math":
# call math function
result = math_function()
elif subject == "science":
# call science function
result = science_function()
elif subject == "history":
# call history function
result = history_function()
return {"result": result}
```
其中,`subject` 参数使用 `Form` 类型注解来获取 HTML 表单数据中的 `subject` 值。根据 `subject` 值的不同,调用相应的函数并返回结果。在函数中,可以使用 HTML 表单数据中的 `subject` 值来获取相应的数据,例如:
```
def math_function():
subject = request.form['subject']
# get math data based on subject value
return math_data
```
相关推荐
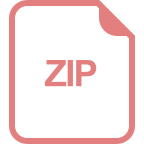














