c# 获取时间段所有月份
时间: 2023-07-27 13:07:33 浏览: 177
你可以通过循环遍历,从起始时间到结束时间,每个月份递增一个月,来获取时间段内所有的月份。
以下是一个示例代码:
```csharp
DateTime start = new DateTime(2021, 1, 1); // 起始时间
DateTime end = new DateTime(2022, 12, 31); // 结束时间
DateTime current = start; // 当前时间
List<DateTime> months = new List<DateTime>(); // 存储所有的月份
while (current <= end)
{
months.Add(current);
current = current.AddMonths(1);
}
foreach (DateTime month in months)
{
Console.WriteLine(month.ToString("yyyy-MM"));
}
```
输出:
```
2021-01
2021-02
2021-03
...
2022-10
2022-11
2022-12
```
这样就可以获取时间段内所有的月份了。
相关问题
c# 获取时间段横跨几个月份
你可以使用 C# 中的 DateTime 和 TimeSpan 类来计算时间段横跨的月份数量。具体步骤如下:
1. 获取时间段的起始日期和结束日期。
2. 使用 TimeSpan 类计算时间段的总天数。
3. 计算起始日期所在月份的第一天,和结束日期所在月份的最后一天。
4. 使用 TimeSpan 类计算起始日期所在月份的天数和结束日期所在月份的天数。
5. 如果时间段起始日期和结束日期在同一月份内,则时间段横跨的月份数量为 1。
6. 否则,计算时间段横跨的完整月份数量。如果起始日期所在月份的第一天早于结束日期所在月份的最后一天,则横跨的月份数量为结束日期所在月份减去起始日期所在月份加 1。
7. 最后,如果起始日期所在月份的天数大于等于结束日期所在月份的天数,则需要减去一月份。
下面是一个示例代码:
```csharp
DateTime startDate = new DateTime(2022, 1, 15);
DateTime endDate = new DateTime(2022, 4, 20);
TimeSpan duration = endDate - startDate;
int totalDays = duration.Days;
DateTime startMonthFirstDay = new DateTime(startDate.Year, startDate.Month, 1);
DateTime endMonthLastDay = new DateTime(endDate.Year, endDate.Month, DateTime.DaysInMonth(endDate.Year, endDate.Month));
int startMonthDays = (startMonthFirstDay.AddMonths(1) - startDate).Days;
int endMonthDays = endDate.Subtract(endMonthLastDay).Days + 1;
int totalMonths = 0;
if (startDate.Month == endDate.Month)
{
totalMonths = 1;
}
else
{
totalMonths = ((endDate.Year - startDate.Year) * 12) + endDate.Month - startDate.Month + 1;
if (startMonthFirstDay < endMonthLastDay)
{
totalMonths--;
}
if (startMonthDays >= endMonthDays)
{
totalMonths--;
}
}
Console.WriteLine("时间段横跨的月份数量为:" + totalMonths);
```
在这个示例代码中,我们假设时间段起始日期为 2022 年 1 月 15 日,结束日期为 2022 年 4 月 20 日。最终输出的结果是时间段横跨的月份数量为 4。
用C# 实现时间段存储器
在C#中,可以使用结构体或类来实现时间段存储器。下面是一个使用类来实现的示例:
```csharp
public class TimePeriod
{
private DateTime startTime;
private DateTime endTime;
public TimePeriod(DateTime start, DateTime end)
{
startTime = start;
endTime = end;
}
public TimeSpan GetDuration()
{
return endTime - startTime;
}
}
```
在上面的示例中,`TimePeriod`类表示一个时间段,它有一个起始时间和一个结束时间。构造函数接受起始时间和结束时间作为参数,并将它们存储在私有字段中。`GetDuration`方法返回时间段的持续时间,即结束时间减去起始时间。
使用示例:
```csharp
DateTime start = new DateTime(2021, 1, 1, 9, 0, 0);
DateTime end = new DateTime(2021, 1, 1, 12, 0, 0);
TimePeriod period = new TimePeriod(start, end);
TimeSpan duration = period.GetDuration();
Console.WriteLine("Duration: " + duration);
```
上面的示例创建了一个时间段对象`period`,起始时间为2021年1月1日9点,结束时间为2021年1月1日12点。然后,使用`GetDuration`方法获取时间段的持续时间,并将其打印输出。
阅读全文
相关推荐
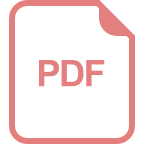
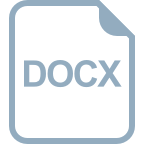
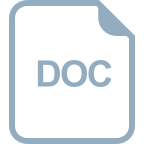













