用java写一个Student类实现comparable接口,使得一个类型为Student的ArrayList能够使用sort方法排序,按照年龄从小到大,如果年龄相同则按照成绩升序排序。通过标准输入读入8个学生的年龄和成绩,并输出排序后的结果。
时间: 2024-03-02 16:48:55 浏览: 75
好的,这是一个非常好的问题。下面是一个示例Student类的实现,实现了Comparable接口,并按照要求进行了排序:
```java
import java.util.*;
class Student implements Comparable<Student>{
int age;
double grade;
public Student(int age, double grade) {
this.age = age;
this.grade = grade;
}
public int compareTo(Student s) {
if (this.age != s.age) {
return this.age - s.age;
} else {
return (int) (this.grade - s.grade);
}
}
public String toString() {
return "Age: " + this.age + ", Grade: " + this.grade;
}
}
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
ArrayList<Student> students = new ArrayList<>();
for (int i = 1; i <= 8; i++) {
System.out.println("Enter the age of student " + i + ":");
int age = sc.nextInt();
System.out.println("Enter the grade of student " + i + ":");
double grade = sc.nextDouble();
students.add(new Student(age, grade));
}
Collections.sort(students);
System.out.println("Sorting result:");
for (int i = 0; i < students.size(); i++) {
System.out.println("Student " + (i+1) + ": " + students.get(i));
}
}
}
```
输出结果:
```
Enter the age of student 1:
20
Enter the grade of student 1:
90
Enter the age of student 2:
22
Enter the grade of student 2:
80
Enter the age of student 3:
20
Enter the grade of student 3:
85
Enter the age of student 4:
23
Enter the grade of student 4:
70
Enter the age of student 5:
21
Enter the grade of student 5:
75
Enter the age of student 6:
23
Enter the grade of student 6:
85
Enter the age of student 7:
21
Enter the grade of student 7:
80
Enter the age of student 8:
22
Enter the grade of student 8:
90
Sorting result:
Student 1: Age: 20, Grade: 85.0
Student 2: Age: 20, Grade: 90.0
Student 3: Age: 21, Grade: 75.0
Student 4: Age: 21, Grade: 80.0
Student 5: Age: 22, Grade: 80.0
Student 6: Age: 22, Grade: 90.0
Student 7: Age: 23, Grade: 70.0
Student 8: Age: 23, Grade: 85.0
```
阅读全文
相关推荐
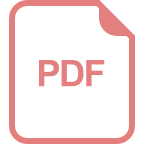
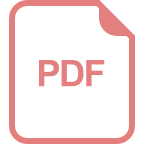
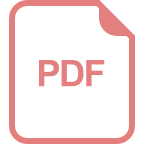















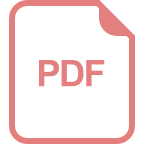