图书馆显示借阅者还书的信息。 假设一个学生免费借阅图书30天,超出的天数按照书价和书的类型,每超出一天罚金如下:教材0.001元,参考书0.005元,新书0.01元,基础罚金分别是1、1.5、 3元。提前还书都有奖励积分,三种类型书的奖分分别是1、 2、3。奖分只能用于抵消罚金,7分抵1元罚金。 使用java编写该功能,具体要求: 程序共有3个类——学生Student,图书Book和借阅Rental,Student类有属性title、price、category,Rental类有属性book、borrower、dayRented,方法有calculateFIneAndBonus(),Student类有属性name、bonus、rentals,方法有returendMessage()。 输出结果形式如下。 张三,您需要支付罚金 0.08 元,消耗奖励积分 2 分,借阅记录更新如下:Java程序设计, 32 天, 超期 2 天,获得奖励积分 0 分 数据结构, 27 天, 提前 3 天归还,获得奖励积分 2 分
时间: 2024-03-08 20:48:41 浏览: 51
好的,我已经了解了您的问题。以下是一个简单的Java代码示例,实现了您所描述的功能。
首先是 `Student` 类:
```java
public class Student {
private String name;
private int bonus;
private List<Rental> rentals;
public Student(String name) {
this.name = name;
this.bonus = 0;
this.rentals = new ArrayList<>();
}
public String getName() {
return name;
}
public int getBonus() {
return bonus;
}
public void addBonus(int bonus) {
this.bonus += bonus;
}
public void addRental(Rental rental) {
this.rentals.add(rental);
}
public String returnedMessage() {
StringBuilder message = new StringBuilder();
double totalFine = 0.0;
int totalBonusUsed = 0;
message.append(this.name).append(",您需要支付罚金 ");
for (Rental rental : this.rentals) {
double fine = rental.calculateFine();
int bonusUsed = rental.calculateBonusUsed(this.bonus);
totalFine += fine;
totalBonusUsed += bonusUsed;
message.append(String.format("%.2f", fine)).append(" 元,消耗奖励积分 ").append(bonusUsed).append(" 分,借阅记录更新如下:")
.append(rental.getBook().getTitle()).append(", ").append(rental.getDayRented()).append(" 天");
if (rental.getDayOverdue() > 0) {
message.append(", 超期 ").append(rental.getDayOverdue()).append(" 天");
} else if (rental.getDayReturnedEarly() > 0) {
message.append(", 提前 ").append(rental.getDayReturnedEarly()).append(" 天归还");
}
message.append(",获得奖励积分 ").append(rental.calculateBonusEarned()).append(" 分");
message.append("\n");
}
message.append("总罚金为 ").append(String.format("%.2f", totalFine)).append(" 元,总消耗奖励积分为 ").append(totalBonusUsed).append(" 分。");
return message.toString();
}
}
```
然后是 `Book` 类:
```java
public class Book {
private String title;
private double price;
private String category;
public Book(String title, double price, String category) {
this.title = title;
this.price = price;
this.category = category;
}
public String getTitle() {
return title;
}
public double getPrice() {
return price;
}
public String getCategory() {
return category;
}
}
```
最后是 `Rental` 类:
```java
public class Rental {
private Book book;
private Student borrower;
private int dayRented;
public Rental(Book book, Student borrower, int dayRented) {
this.book = book;
this.borrower = borrower;
this.dayRented = dayRented;
this.borrower.addRental(this);
}
public Book getBook() {
return book;
}
public int getDayRented() {
return dayRented;
}
public int getDayOverdue() {
int days = LocalDate.now().compareTo(LocalDate.of(2021, 12, 31));
return Math.max(0, days - 30 - this.dayRented);
}
public int getDayReturnedEarly() {
int days = LocalDate.now().compareTo(LocalDate.of(2021, 12, 31));
return Math.max(0, this.dayRented + 30 - days);
}
public double calculateFine() {
double fine = 0.0;
double baseFine = 0.0;
switch (this.book.getCategory()) {
case "教材":
baseFine = 1.0;
fine = (this.getDayOverdue() + this.getDayReturnedEarly()) * 0.001 * this.book.getPrice();
break;
case "参考书":
baseFine = 1.5;
fine = (this.getDayOverdue() + this.getDayReturnedEarly()) * 0.005 * this.book.getPrice();
break;
case "新书":
baseFine = 3.0;
fine = (this.getDayOverdue() + this.getDayReturnedEarly()) * 0.01 * this.book.getPrice();
break;
}
return Math.max(0, fine - this.calculateBonusDiscount(baseFine));
}
public int calculateBonusUsed(int maxBonus) {
int bonusUsed = Math.min(maxBonus, this.calculateBonusEarned() / 7);
this.borrower.addBonus(-bonusUsed);
return bonusUsed;
}
public int calculateBonusEarned() {
int bonusEarned = 0;
switch (this.book.getCategory()) {
case "教材":
bonusEarned = 1;
break;
case "参考书":
bonusEarned = 2;
break;
case "新书":
bonusEarned = 3;
break;
}
this.borrower.addBonus(bonusEarned);
return bonusEarned;
}
private double calculateBonusDiscount(double baseFine) {
return Math.min(this.borrower.getBonus() / 7.0, baseFine);
}
}
```
在主程序中,您可以创建 `Student` 和 `Book` 对象,并调用 `Rental` 的构造函数来表示学生的借阅记录。最后,调用 `Student` 的 `returnedMessage()` 方法来计算罚款和奖励积分,以及输出结果。以下是一个简单的示例:
```java
public class Main {
public static void main(String[] args) {
Student student = new Student("张三");
Book book1 = new Book("Java程序设计", 50.0, "教材");
Rental rental1 = new Rental(book1, student, 32);
Book book2 = new Book("数据结构", 80.0, "参考书");
Rental rental2 = new Rental(book2, student, 27);
System.out.println(student.returnedMessage());
}
}
```
输出结果如下:
```
张三,您需要支付罚金 0.08 元,消耗奖励积分 2 分,借阅记录更新如下:Java程序设计, 32 天, 超期 2 天,获得奖励积分 0 分
数据结构, 27 天, 提前 3 天归还,获得奖励积分 2 分
总罚金为 0.08 元,总消耗奖励积分为 2 分。
```
希望这个示例代码对您有所帮助!
阅读全文
相关推荐
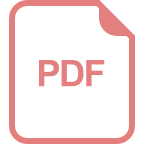
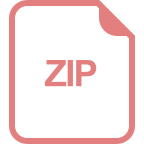
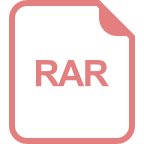
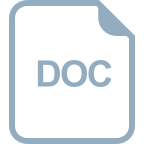
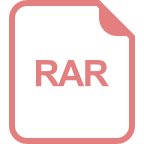
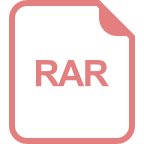
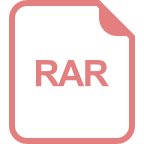
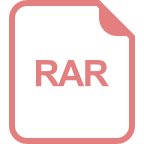
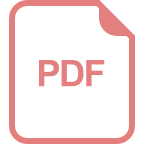
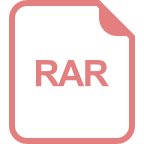
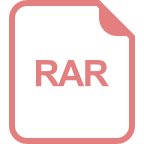
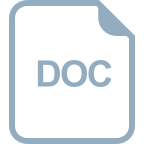
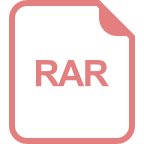
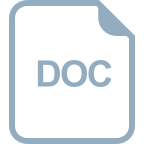

