如何解决将String值反序列化为`java.lang.reflect.Array`类型时遇到的问题:Cannot deserialize value of type `java.lang.reflect.Array` from Array value
时间: 2024-12-13 09:10:42 浏览: 8
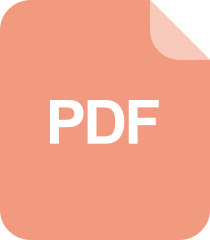
Android Caused by: java.lang.ClassNotFoundException解决办法
当你尝试将一个包含Array类型的字符串反序列化到`java.lang.reflect.Array`,而实际上接收到的是数组值时,可能会遇到这个问题。这是因为序列化和反序列化默认处理的是具体的数组类型,而不是`Array`类本身。
要解决这个问题,你可以按照以下步骤操作:
1. **自定义序列化器**:创建一个自定义的`ObjectOutputStream`或`ObjectInputStream`的适配器,比如实现`Serializable`接口的`ArraySerializer`或`ArrayDeserializer`。这个类需要有一个方法来解析输入的Array字符串并将其转换为实际的数组实例。
```java
public class ArraySerializer extends ObjectOutputStream {
@Override
public void writeObject(Object obj) throws IOException {
if (obj instanceof String && Array.class.isInstance(obj)) {
// 解析字符串得到数组类型和元素列表
Class<?> componentType = getComponentTypeFromString((String) obj);
List<Object> arrayElements = ...; // 根据字符串构建数组元素
writeArray(arrayElements, componentType);
} else {
super.writeObject(obj);
}
}
private void writeArray(List<Object> elements, Class<?> componentType) throws IOException {
// 实现将List转换为实际数组并序列化
Array.arrayCopy(elements.toArray(), 0, out, 0, elements.size());
}
}
public class ArrayDeserializer extends ObjectInputStream {
@Override
protected Object readUnshared() throws IOException, ClassNotFoundException {
return isWriting() ? readArray() : super.readUnshared();
}
private Array readArray() throws IOException {
// 读取序列化的数组数据,并构造对应的Array对象
String arrayDesc = readUTF(); // 读取描述符(如"[Ljava/lang/String;")
String[] components = arrayDesc.substring(1, -1).split(","); // 提取组件类型
Class<?> componentType = Class.forName(components[0].trim());
int length = readInt(); // 读取数组长度
return Array.newInstance(componentType, length);
}
}
```
2. **使用`@JsonDeserialize`和`@JsonSerialize`注解**:如果你正在使用JSON库(如Jackson),可以利用这些注解来指定自定义的反序列化和序列化逻辑。
```java
@JsonDeserialize(using = ArrayDeserializer.class)
@JsonSerialize(using = ArraySerializer.class)
private Object myArrayField;
```
3. **手动转换**:在反序列化后,检查是否是`Array`类型,然后转换成具体的数组实例。
```java
String serializedArrayStr = ...;
Object unserialized = yourDeserializationMethod(serializedArrayStr);
if (unserialized instanceof String && Array.class.isInstance(unserialized)) {
Class<?> componentType = ...; // 获取组件类型
unserialized = Array.getArrayInstance(componentType, ((String) unserialized).split("\\[")[1].split("\\]")[0].split(",")); // 转换为数组
}
```
阅读全文
相关推荐
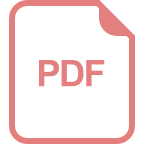

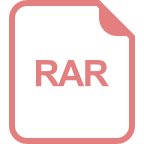
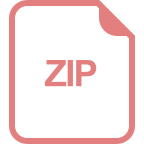
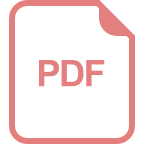
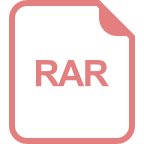
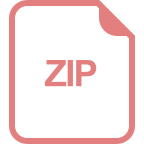
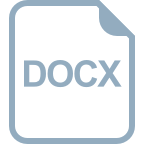
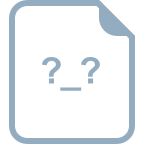
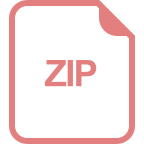
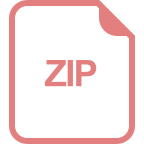
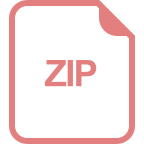
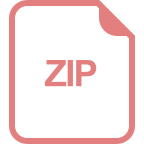
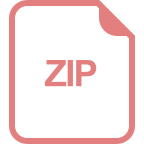
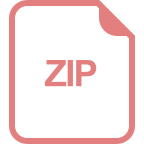
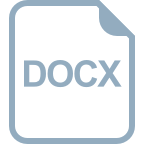
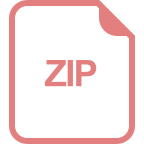