贝塞尔曲线轮廓图偏移
时间: 2024-12-26 17:17:06 浏览: 4
### 贝塞尔曲线轮廓图偏移算法实现
对于贝塞尔曲线轮廓图的偏移操作,通常涉及几何变换和数值计算。具体来说,在给定一条贝塞尔曲线的情况下,为了创建其平行线或轮廓线,需要考虑法向量方向上的位移。
#### 法向量计算
每一点处的法向量可以通过求解该点切线的方向并旋转90度获得。对于参数化的三次贝塞尔曲线 \( B(t) \),其中 \( t ∈ [0, 1] \),可以先通过导数得到任意位置的切矢:
\[ B'(t)=3(1-t)^2(P_1-P_0)+6(1-t)t(P_2-P_1)+3t^2(P_3-P_2) \]
接着利用叉积来获取垂直于这条直线的新向量作为法向量 N(t)[^1]。
```python
import numpy as np
def bezier_derivative(p0, p1, p2, p3, t):
"""Calculate derivative of cubic Bezier at parameter value `t`."""
return (
3 * (1 - t)**2 * (p1 - p0) +
6 * (1 - t) * t * (p2 - p1) +
3 * t**2 * (p3 - p2)
)
def normalize(v):
norm = np.linalg.norm(v)
if norm == 0:
return v
return v / norm
def normal_vector(control_points, t):
"""Compute normalized normal vector at point defined by parametric variable `t`"""
deriv = bezier_derivative(*control_points, t)
perp = np.array([-deriv[1], deriv[0]]) # Perpendicular to tangent
return normalize(perp)
```
#### 偏移距离应用
一旦有了法向量,则可以在每个采样点上沿此方向移动指定的距离 d 来形成新的边界。需要注意的是当两条相邻边相交时可能会出现问题;因此实际应用中往往还需要额外处理这些特殊情况以确保最终图形不会自交。
```python
def offset_bezier(control_points, distance, num_samples=100):
ts = np.linspace(0, 1, num_samples)
new_control_points = []
for t in ts:
pos = sum((1-t)**i * t**(3-i) * comb(3,i) * control_points[i] for i in range(4))
nrm = normal_vector(control_points, t)
displaced_point = pos + nrm * distance
new_control_points.append(displaced_point.tolist())
return new_control_points
```
上述代码片段展示了如何基于原始控制点集构建一个新的、经过偏移后的贝塞尔曲线序列。
阅读全文
相关推荐










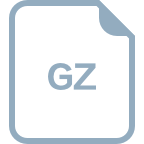

