python 创建字典 增加值
时间: 2024-04-23 19:20:18 浏览: 11
在Python中,可以使用大括号{}来创建字典。字典是一种无序的键值对集合,每个键值对之间用逗号分隔。键必须是唯一的,而值可以是任意类型的数据。
要创建一个空字典,可以直接使用大括号{},如下所示:
```
my_dict = {}
```
如果要创建一个非空字典,可以在大括号内部使用键值对来初始化字典,如下所示:
```
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
```
要向字典中添加新的键值对,可以使用以下方法:
```
my_dict['new_key'] = 'new_value'
```
这将在字典中添加一个新的键值对。
如果要更新已存在的键的值,可以直接通过键来访问并赋予新的值,如下所示:
```
my_dict['existing_key'] = 'updated_value'
```
这将更新字典中已存在键的值。
相关问题
Python中字典增加元素
可以使用字典的键来增加元素。如果键不存在,它将被创建并分配一个值。如果键已经存在,则值将被更新。
示例代码:
```
my_dict = {'name': 'Alice', 'age': 25}
my_dict['city'] = 'New York'
print(my_dict) # 输出:{'name': 'Alice', 'age': 25, 'city': 'New York'}
```
在上面的代码中,我们使用 `my_dict['city'] = 'New York'` 将新元素添加到字典中。现在,字典包含三个键值对:`name: Alice`,`age: 25` 和 `city: New York`。
python 根据键增加值
要在 Python 中根据键增加值,你可以使用字典(dictionary)的方法。字典是一种可变的数据类型,它由键-值对(key-value pairs)组成。
下面是一种常见的方法来根据键增加值:
```python
# 创建一个空字典
my_dict = {}
# 添加键-值对
my_dict['key'] = 'value'
# 增加键的值
my_dict['key'] += ' additional value'
print(my_dict['key']) # 输出:value additional value
```
在上面的例子中,我们首先创建了一个空字典 `my_dict`。然后,我们使用`['key']`来指定要增加值的键,然后使用赋值运算符 `=` 来给这个键赋值。最后,我们可以通过引用键来访问该值并进行操作。
希望这可以帮助到你!如有其他问题,请随时提问。
相关推荐
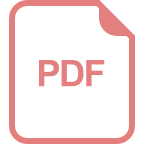
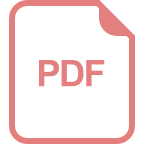
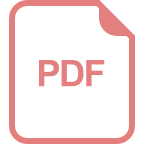
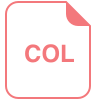
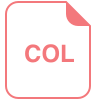
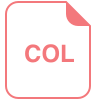
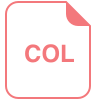
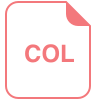







