objectarx中采用 Douglas-Peucker 算法进行多段线简化,使用C++实现,并附详细代码,不能使用setSpline()和simplify()
时间: 2024-02-23 09:58:00 浏览: 74
好的,我了解了您的要求。下面是一个简单的C++实现,使用Douglas-Peucker算法对多段线进行简化:
```cpp
#include <iostream>
#include <vector>
#include <cmath>
using namespace std;
struct Point {
double x, y;
Point(double x = 0, double y = 0) : x(x), y(y) {}
};
// 计算点p到线段AB的距离
double distToSegment(Point p, Point A, Point B) {
double d = (B - A).x * (p - A).y - (B - A).y * (p - A).x;
return fabs(d) / (B - A).length();
}
// Douglas-Peucker算法简化多段线
void simplify(vector<Point>& points, double tolerance) {
if (points.size() < 3) return;
vector<Point> result;
double maxDist = 0;
int maxIndex = 0;
for (int i = 1; i < points.size() - 1; i++) {
double d = distToSegment(points[i], points[0], points[points.size() - 1]);
if (d > maxDist) {
maxDist = d;
maxIndex = i;
}
}
if (maxDist > tolerance) {
vector<Point> left(points.begin(), points.begin() + maxIndex + 1);
vector<Point> right(points.begin() + maxIndex, points.end());
simplify(left, tolerance);
simplify(right, tolerance);
result.insert(result.end(), left.begin(), left.end() - 1);
result.insert(result.end(), right.begin(), right.end());
points = result;
}
}
int main() {
vector<Point> points = {Point(0, 0), Point(1, 1), Point(2, 2), Point(3, 1), Point(4, 0)};
simplify(points, 0.5);
for (auto& p : points) {
cout << "(" << p.x << ", " << p.y << ")" << endl;
}
return 0;
}
```
上面的代码中,我们定义了一个`Point`结构体来表示二维坐标系中的点,同时我们还定义了一个`distToSegment`函数来计算一个点到线段的距离。接着,我们实现了一个`simplify`函数来进行多段线的简化,其中`tolerance`参数表示简化的容差。最后,我们在`main`函数中测试了一下`simplify`函数的效果。
需要注意的是,上面的代码中并没有使用`setSpline`和`simplify`函数,而是自己实现了一个简单的Douglas-Peucker算法来进行多段线的简化。
阅读全文
相关推荐
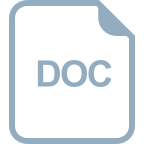
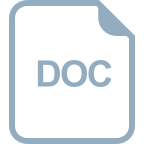
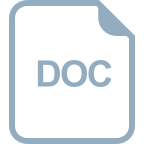



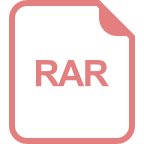
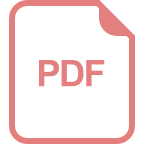
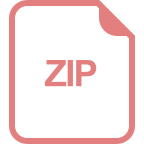
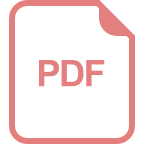
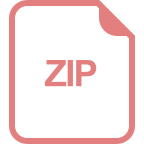
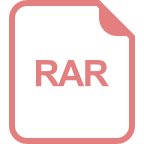
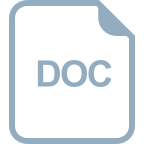
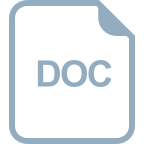
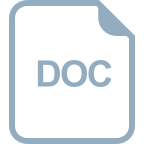

