设计一个学生类CStudent,其数据成员是学生的学号、姓名、数学、外语、计算机课程成绩。 建立学生对象的数组并输入相关信息,打印所有学生信息。 查找出学号为n的学生的信息。 学生类增加一个成员方法:求平均分 测试类中将学生按照平均分排序输出用java实现
时间: 2023-12-16 11:51:52 浏览: 23
好的,以下是实现代码:
```java
import java.util.Arrays;
import java.util.Scanner;
class CStudent {
private String id; // 学号
private String name; // 姓名
private double mathScore; // 数学成绩
private double englishScore; // 外语成绩
private double computerScore; // 计算机成绩
public CStudent(String id, String name, double mathScore, double englishScore, double computerScore) {
this.id = id;
this.name = name;
this.mathScore = mathScore;
this.englishScore = englishScore;
this.computerScore = computerScore;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public double getMathScore() {
return mathScore;
}
public double getEnglishScore() {
return englishScore;
}
public double getComputerScore() {
return computerScore;
}
public double getAvgScore() {
return (mathScore + englishScore + computerScore) / 3.0;
}
@Override
public String toString() {
return "学号:" + id + ",姓名:" + name + ",数学成绩:" + mathScore +
",外语成绩:" + englishScore + ",计算机成绩:" + computerScore;
}
}
public class Test {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 输入学生个数
System.out.print("请输入学生个数:");
int n = scanner.nextInt();
// 创建学生对象数组
CStudent[] students = new CStudent[n];
// 输入学生信息
for (int i = 0; i < n; i++) {
System.out.println("请输入第" + (i + 1) + "个学生的信息:");
System.out.print("学号:");
String id = scanner.next();
System.out.print("姓名:");
String name = scanner.next();
System.out.print("数学成绩:");
double mathScore = scanner.nextDouble();
System.out.print("外语成绩:");
double englishScore = scanner.nextDouble();
System.out.print("计算机成绩:");
double computerScore = scanner.nextDouble();
students[i] = new CStudent(id, name, mathScore, englishScore, computerScore);
}
// 打印学生信息
System.out.println("所有学生信息:");
for (CStudent student : students) {
System.out.println(student);
}
// 查找学号为n的学生信息
System.out.print("请输入要查找的学号:");
String targetId = scanner.next();
for (CStudent student : students) {
if (student.getId().equals(targetId)) {
System.out.println("找到了该学生,信息如下:");
System.out.println(student);
}
}
// 按照平均分排序并输出
Arrays.sort(students, (s1, s2) -> Double.compare(s2.getAvgScore(), s1.getAvgScore()));
System.out.println("按照平均分排序后的学生信息:");
for (CStudent student : students) {
System.out.println(student);
}
}
}
```
希望我的回答能够帮助到你!
相关推荐
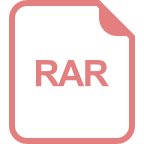
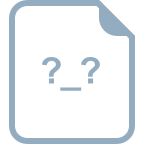
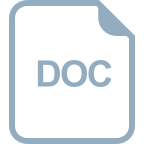














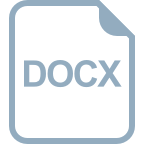
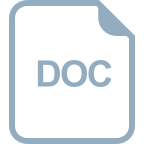