5、设计一个CStudent(学生)类,并使CStudent类具有以下特点: 1)该类有学生姓名学号和几门课程的成绩; 2)学生全部信息由键盘输入,以提高程序的适应性; 3)通过成员函数统计学生平均成绩,当课程数量增加时,成员函数无须修改仍可以求取平均成绩; 4)输出学生的基本信息、各科成绩与平均成绩; 5)学生对象的定义采用对象数组实现
时间: 2023-05-29 11:06:15 浏览: 207
#include<iostream>
using namespace std;
class CStudent{
private:
string name; //学生姓名
int id; //学号
int numOfCourse; //课程数量
int* score; //指向动态数组的指针,存储各科成绩
public:
CStudent(){ //构造函数,初始化成员变量
name = "";
id = 0;
numOfCourse = 0;
score = NULL;
}
~CStudent(){ //析构函数,释放动态数组的内存空间
if(score != NULL){
delete[] score;
score = NULL;
}
}
void inputInfo(){ //输入学生信息和各科成绩
cout << "请输入学生姓名:";
cin >> name;
cout << "请输入学号:";
cin >> id;
cout << "请输入课程数量:";
cin >> numOfCourse;
score = new int[numOfCourse]; //动态分配数组内存空间
cout << "请输入各科成绩:";
for(int i=0; i<numOfCourse; i++){
cin >> score[i];
}
}
float getAverageScore(){ //计算平均成绩
float sum = 0;
for(int i=0; i<numOfCourse; i++){
sum += score[i];
}
return sum/numOfCourse;
}
void outputInfo(){ //输出学生信息、各科成绩和平均成绩
cout << "学生姓名:" << name << endl;
cout << "学号:" << id << endl;
cout << "各科成绩:";
for(int i=0; i<numOfCourse; i++){
cout << score[i] << " ";
}
cout << endl;
cout << "平均成绩:" << getAverageScore() << endl;
}
};
int main(){
int n;
cout << "请输入学生人数:";
cin >> n;
CStudent* students = new CStudent[n]; //定义对象数组
for(int i=0; i<n; i++){
students[i].inputInfo(); //输入学生信息和各科成绩
}
for(int i=0; i<n; i++){
students[i].outputInfo(); //输出学生信息、各科成绩和平均成绩
}
delete[] students; //释放对象数组的内存空间
return 0;
}
阅读全文
相关推荐
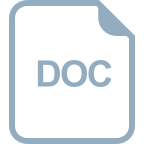
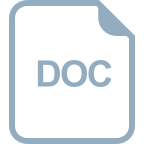
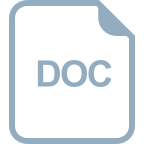




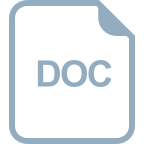




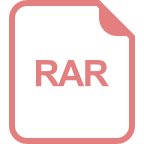
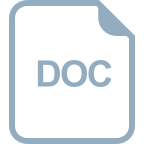


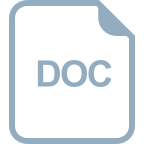
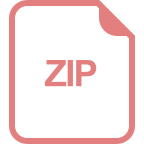