最大熵阈值分割Python
时间: 2023-07-07 09:36:13 浏览: 118
最大熵阈值分割是一种常用的图像分割方法之一,可以用于将一幅图像分割成多个区域。下面是一个简单的最大熵阈值分割的Python实现:
```python
import numpy as np
from PIL import Image
def max_entropy_threshold(image):
# 将图像转为灰度图
image = image.convert('L')
# 计算图像的直方图
hist, bins = np.histogram(image, bins=256, range=(0, 255))
# 归一化直方图
hist = hist / np.sum(hist)
# 计算累计直方图
cum_hist = np.cumsum(hist)
# 初始化最大熵和阈值
max_entropy = 0
threshold = 0
# 遍历每个可能的阈值
for t in range(256):
# 计算背景和前景的概率分布
hist_bg = hist[:t]
hist_fg = hist[t:]
# 计算背景和前景的累计概率分布
cum_hist_bg = cum_hist[t]
cum_hist_fg = 1 - cum_hist_bg
# 计算背景和前景的熵
entropy_bg = -np.sum(hist_bg * np.log(hist_bg + 1e-6))
entropy_fg = -np.sum(hist_fg * np.log(hist_fg + 1e-6))
# 计算总的熵
entropy = cum_hist_bg * entropy_bg + cum_hist_fg * entropy_fg
# 更新最大熵和阈值
if entropy > max_entropy:
max_entropy = entropy
threshold = t
# 返回阈值
return threshold
# 加载图像
image = Image.open('lena.png')
# 计算最大熵阈值
threshold = max_entropy_threshold(image)
# 对图像进行二值化
image = image.convert('L').point(lambda x: 255 if x > threshold else 0)
# 显示二值化后的图像
image.show()
```
这个实现中,我们首先将图像转为灰度图,然后计算图像的直方图和累计直方图。接着遍历每个可能的阈值,计算背景和前景的概率分布、累计概率分布和熵,并更新最大熵和阈值。最后根据阈值对图像进行二值化,并显示二值化后的图像。
相关推荐
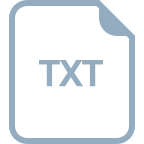














