ExcelUtils.read
时间: 2023-08-16 20:10:16 浏览: 125
`ExcelUtils.read()` 是一个自定义的方法,用于读取 Excel 文件并将其内容转换成 List<Map<String, String>> 的格式。该方法的具体实现可能与不同的项目和库有所不同,以下是一种可能的实现方式:
```java
public static List<Map<String, String>> read(String filePath) {
File file = new File(filePath);
if (!file.exists() || !file.isFile()) {
return null;
}
Workbook workbook = null;
try {
workbook = WorkbookFactory.create(file);
Sheet sheet = workbook.getSheetAt(0); // 获取第一个工作表
int rowCount = sheet.getLastRowNum() + 1;
Row firstRow = sheet.getRow(0); // 获取第一行,通常作为表头
int columnCount = firstRow.getLastCellNum();
List<Map<String, String>> result = new ArrayList<>();
for (int i = 1; i < rowCount; i++) { // 从第二行开始遍历
Row currentRow = sheet.getRow(i);
Map<String, String> rowMap = new HashMap<>();
for (int j = 0; j < columnCount; j++) {
Cell cell = currentRow.getCell(j, Row.MissingCellPolicy.CREATE_NULL_AS_BLANK);
String cellValue = getCellValue(cell);
rowMap.put(getCellKey(firstRow.getCell(j)), cellValue);
}
result.add(rowMap);
}
return result;
} catch (Exception e) {
e.printStackTrace();
return null;
} finally {
if (workbook != null) {
try {
workbook.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
private static String getCellValue(Cell cell) {
if (cell.getCellType() == CellType.NUMERIC) {
return String.valueOf(cell.getNumericCellValue());
} else {
return cell.getStringCellValue();
}
}
private static String getCellKey(Cell cell) {
return cell.getStringCellValue().trim();
}
```
该方法首先会检查文件是否存在,如果文件不存在或者不是一个文件,就会返回 `null`。然后,它会使用 Apache POI 库打开 Excel 文件,并遍历每一行,将每一行转换成一个 Map<String, String> 对象,并将所有的 Map 对象添加到一个列表中,最终返回该列表。
阅读全文
相关推荐
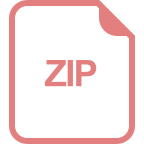
















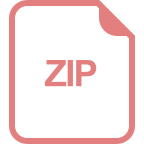