请用python tkinter库写一个双人贪吃蛇游戏
时间: 2023-11-18 15:05:58 浏览: 84
好的,以下是使用Python的Tkinter库编写双人贪吃蛇游戏的代码示例:
```python
import tkinter as tk
import random
# 游戏界面的宽度和高度
WIDTH = 400
HEIGHT = 400
# 蛇的身体长度和速度
SNAKE_SIZE = 20
SNAKE_SPEED = 100
class SnakeGame(tk.Tk):
def __init__(self):
tk.Tk.__init__(self)
self.canvas = tk.Canvas(self, width=WIDTH, height=HEIGHT)
self.canvas.pack()
# 初始化蛇的位置和方向
self.snake = [(100, 100), (80, 100), (60, 100)]
self.direction = "Right"
# 初始化食物的位置
self.food = self._create_food()
# 绑定键盘事件
self.bind("<Key>", self._on_key_press)
# 开始游戏循环
self._game_loop()
def _create_food(self):
x = random.randint(1, (WIDTH - SNAKE_SIZE) // SNAKE_SIZE) * SNAKE_SIZE
y = random.randint(1, (HEIGHT - SNAKE_SIZE) // SNAKE_SIZE) * SNAKE_SIZE
return x, y
def _draw_snake(self):
self.canvas.delete("snake")
for x, y in self.snake:
self.canvas.create_rectangle(x, y, x + SNAKE_SIZE, y + SNAKE_SIZE, fill="green", tag="snake")
def _draw_food(self):
x, y = self.food
self.canvas.create_rectangle(x, y, x + SNAKE_SIZE, y + SNAKE_SIZE, fill="red", tag="food")
def _move_snake(self):
head_x, head_y = self.snake[0]
if self.direction == "Up":
new_head = (head_x, head_y - SNAKE_SIZE)
elif self.direction == "Down":
new_head = (head_x, head_y + SNAKE_SIZE)
elif self.direction == "Left":
new_head = (head_x - SNAKE_SIZE, head_y)
else:
new_head = (head_x + SNAKE_SIZE, head_y)
self.snake.insert(0, new_head)
self.snake.pop()
def _check_collision(self):
head_x, head_y = self.snake[0]
if (
head_x < 0
or head_x >= WIDTH
or head_y < 0
or head_y >= HEIGHT
or (head_x, head_y) in self.snake[1:]
):
return True
if (head_x, head_y) == self.food:
self.snake.append((0, 0))
self.food = self._create_food()
return False
def _on_key_press(self, event):
if event.keysym == "Up" and self.direction != "Down":
self.direction = "Up"
elif event.keysym == "Down" and self.direction != "Up":
self.direction = "Down"
elif event.keysym == "Left" and self.direction != "Right":
self.direction = "Left"
elif event.keysym == "Right" and self.direction != "Left":
self.direction = "Right"
def _game_loop(self):
if not self._check_collision():
self._move_snake()
self._draw_snake()
self._draw_food()
self.after(SNAKE_SPEED, self._game_loop)
else:
self.canvas.create_text(
WIDTH // 2,
HEIGHT // 2,
text="Game Over",
font=("Helvetica", 24),
fill="red",
tag="gameover",
)
game = SnakeGame()
game.mainloop()
```
这个代码使用Tkinter库创建了一个窗口,游戏界面大小为400x400像素。蛇的身体由矩形组成,随着方向键的按下而移动。食物是一个红色的方块,当蛇吃掉食物时,身体长度增加。如果蛇撞到边界或自己的身体,游戏结束。
阅读全文
相关推荐
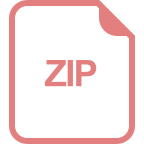
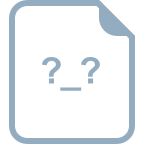
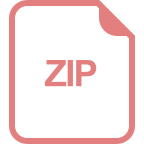















