c语言贪吃蛇游戏代码mac
时间: 2023-10-14 08:30:08 浏览: 193
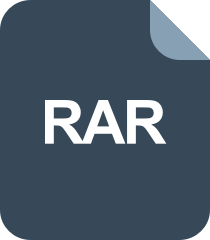
C语言贪吃蛇游戏代码
以下是一个简单的 C 语言贪吃蛇游戏代码,适用于 macOS 系统:
```c
#include <stdio.h>
#include <stdlib.h>
#include <termios.h>
#include <unistd.h>
#include <fcntl.h>
#include <time.h>
#define ROW 20
#define COL 40
#define UP 'w'
#define DOWN 's'
#define LEFT 'a'
#define RIGHT 'd'
int speed = 100000;
char map[ROW][COL];
struct Snake {
int x;
int y;
struct Snake *next;
};
struct Food {
int x;
int y;
};
struct Snake *head, *tail;
struct Food food;
struct termios oldt, newt;
void init() {
int i, j;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
if (i == 0 || i == ROW - 1 || j == 0 || j == COL - 1) {
map[i][j] = '#';
} else {
map[i][j] = ' ';
}
}
}
head = (struct Snake*) malloc(sizeof(struct Snake));
head->x = ROW / 2;
head->y = COL / 2;
head->next = NULL;
tail = head;
map[head->x][head->y] = '*';
food.x = rand() % (ROW - 2) + 1;
food.y = rand() % (COL - 2) + 1;
map[food.x][food.y] = '@';
}
void draw() {
int i, j;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
printf("%c", map[i][j]);
}
printf("\n");
}
}
void update() {
int ch = getchar();
struct Snake *new_head = (struct Snake*) malloc(sizeof(struct Snake));
new_head->next = head;
switch (ch) {
case UP:
new_head->x = head->x - 1;
new_head->y = head->y;
break;
case DOWN:
new_head->x = head->x + 1;
new_head->y = head->y;
break;
case LEFT:
new_head->x = head->x;
new_head->y = head->y - 1;
break;
case RIGHT:
new_head->x = head->x;
new_head->y = head->y + 1;
break;
default:
new_head->x = head->x - 1;
new_head->y = head->y;
break;
}
if (new_head->x == food.x && new_head->y == food.y) {
tail->next = new_head;
tail = new_head;
map[tail->x][tail->y] = '*';
food.x = rand() % (ROW - 2) + 1;
food.y = rand() % (COL - 2) + 1;
map[food.x][food.y] = '@';
speed -= 5000;
} else {
map[tail->x][tail->y] = ' ';
tail->x = new_head->x;
tail->y = new_head->y;
map[tail->x][tail->y] = '*';
struct Snake *p = head;
while (p->next != tail) {
p = p->next;
}
free(new_head);
p->next = NULL;
}
head = tail;
}
void gameover() {
printf("Game Over!\n");
tcsetattr(STDIN_FILENO, TCSANOW, &oldt);
exit(0);
}
int main() {
srand(time(NULL));
init();
tcgetattr(STDIN_FILENO, &oldt);
newt = oldt;
newt.c_lflag &= ~(ICANON | ECHO);
tcsetattr(STDIN_FILENO, TCSANOW, &newt);
while (1) {
draw();
usleep(speed);
update();
if (head->x == 0 || head->x == ROW - 1 || head->y == 0 || head->y == COL - 1) {
gameover();
}
struct Snake *p = head->next;
while (p != NULL) {
if (head->x == p->x && head->y == p->y) {
gameover();
}
p = p->next;
}
}
return 0;
}
```
该代码使用了标准库中的头文件 `<stdio.h>`、`<stdlib.h>`、`<termios.h>`、`<unistd.h>`、`<fcntl.h>` 和 `<time.h>`。
注意:由于 macOS 系统没有 kbhit 函数,所以该代码使用了 termios.h 头文件中的 tcsetattr 和 tcgetattr 函数来实现键盘输入的非阻塞。
阅读全文
相关推荐
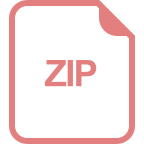
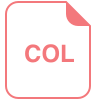


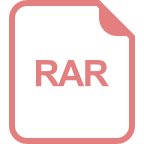
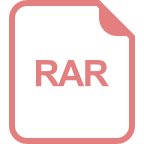
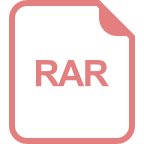
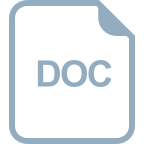
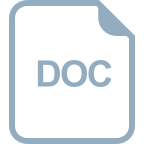
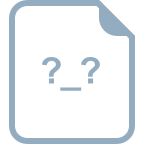
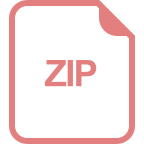
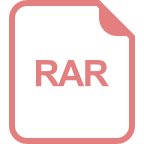
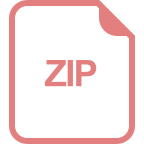
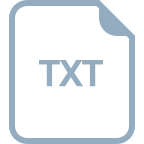
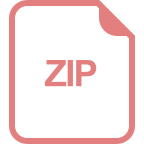