文件系统拷贝功能模拟
时间: 2023-07-19 12:07:22 浏览: 56
实现文件系统拷贝功能可以采用递归的方式遍历源文件夹,将文件和文件夹复制到目标文件夹中。具体实现过程如下:
1. 接收源文件夹路径和目标文件夹路径作为输入参数。
2. 判断源文件夹是否存在,如果不存在则退出程序或者报错提示。
3. 判断目标文件夹是否存在,如果不存在则创建目录。
4. 遍历源文件夹中的所有文件和文件夹,对于每一个文件或者文件夹,分别进行以下操作:
1. 如果是文件,则将该文件复制到目标文件夹中。
2. 如果是文件夹,则在目标文件夹中创建一个同名的文件夹,并将该文件夹下所有文件和文件夹递归复制到新创建的文件夹中。
5. 复制完成后,输出复制结果。
实现过程中需要注意以下几点:
1. 需要判断源文件夹和目标文件夹是否相同,如果相同则不能进行复制。
2. 复制过程中需要处理文件重名的情况,可以在文件名后面加上数字进行区分。
3. 复制过程中需要处理文件权限和属性的问题,例如文件权限、修改时间等信息需要保持一致。
4. 需要处理文件拷贝过程中可能出现的异常情况,例如读写错误、磁盘空间不足等问题。
示例代码如下:
```python
import os
import shutil
def copy_folder(src_folder, dst_folder):
if not os.path.exists(src_folder):
raise Exception("Source folder does not exist!")
if os.path.abspath(src_folder) == os.path.abspath(dst_folder):
raise Exception("Source folder and destination folder are the same!")
if not os.path.exists(dst_folder):
os.makedirs(dst_folder)
for item in os.listdir(src_folder):
src_item = os.path.join(src_folder, item)
dst_item = os.path.join(dst_folder, item)
if os.path.isfile(src_item):
if os.path.exists(dst_item):
i = 1
while True:
new_dst_item = os.path.join(dst_folder, f"{os.path.splitext(item)[0]}_{i}{os.path.splitext(item)[1]}")
if not os.path.exists(new_dst_item):
dst_item = new_dst_item
break
i += 1
shutil.copy2(src_item, dst_item)
elif os.path.isdir(src_item):
copy_folder(src_item, dst_item)
print(f"{src_folder} copied to {dst_folder} successfully!")
```
相关推荐
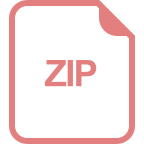
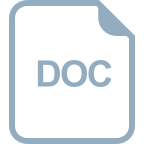
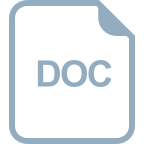












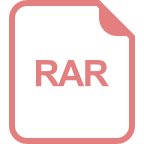
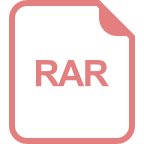
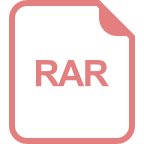