public Connection getConn() { try { String d = new Date().toLocaleString().substring(0, 9); Class.forName("com.mysql.jdbc.Driver"); String dburl = (String) config.get("dburl"); conn = DriverManager.getConnection(dburl, "root", "123456"); } catch (Exception e) { e.printStackTrace(); } return conn; }
时间: 2024-04-28 18:21:30 浏览: 10
这段代码看起来是获取数据库连接的方法,使用了JDBC连接数据库。其中使用了MySQL的JDBC驱动程序,连接字符串从配置文件中读取。同时,连接的用户名为"root",密码为"123456"。该方法返回获取到的数据库连接对象。不过,这段代码并不够安全,用户名和密码应该从加密的配置文件中读取,并且应该使用连接池技术来保证连接的有效性和避免资源浪费。
相关问题
public List<Visitor> findAll() { conn = JDBCUtils.getConn(); Visitor visitor = null; String sql = "SELECT * FROM blacklist"; try { ps = conn.prepareStatement(sql); rs = ps.executeQuery(); List<Visitor> list = new ArrayList<>(); while (rs.next()) { // 通过字段检索 int pid = rs.getInt("id"); String name = rs.getString("name"); String phone = rs.getString("phone"); String reason = rs.getString("reason"); visitor = new Visitor(pid, name, phone, reason); list.add(visitor); } return list; } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } JDBCUtils.close(conn, ps, rs); return null; } 详细解释这段代码中每一句代码的意思
这段代码是一个使用 JDBC 访问数据库的示例。以下是每一句代码的解释:
```java
public List<Visitor> findAll() {
conn = JDBCUtils.getConn(); // 获取数据库连接对象
Visitor visitor = null; // 定义一个 Visitor 对象,用于存储每一行数据
String sql = "SELECT * FROM blacklist"; // 定义 SQL 查询语句
try {
ps = conn.prepareStatement(sql); // 创建 PreparedStatement 对象
rs = ps.executeQuery(); // 执行查询,返回 ResultSet 对象
List<Visitor> list = new ArrayList<>(); // 创建一个 Visitor 对象的列表,用于存储查询结果
while (rs.next()) { // 遍历查询结果
// 通过字段检索
int pid = rs.getInt("id"); // 获取 id 字段的值
String name = rs.getString("name"); // 获取 name 字段的值
String phone = rs.getString("phone"); // 获取 phone 字段的值
String reason = rs.getString("reason"); // 获取 reason 字段的值
// 将查询结果封装成一个 Visitor 对象,并加入列表中
visitor = new Visitor(pid, name, phone, reason);
list.add(visitor);
}
return list; // 返回查询结果列表
} catch (SQLException e) { // 处理 SQL 异常
e.printStackTrace();
}
JDBCUtils.close(conn, ps, rs); // 关闭数据库连接、PreparedStatement 对象和 ResultSet 对象
return null;
}
```
在上面的代码中,`JDBCUtils` 是一个自定义的 JDBC 工具类,其中包含了获取数据库连接、关闭数据库连接等常用操作的方法。以下是 `JDBCUtils.getConn()` 和 `JDBCUtils.close()` 方法的代码示例:
```java
public class JDBCUtils {
private static final String USERNAME = "root";
private static final String PASSWORD = "root";
private static final String DRIVER = "com.mysql.jdbc.Driver";
private static final String URL = "jdbc:mysql://localhost:3306/test";
// 获取数据库连接对象
public static Connection getConn() {
Connection conn = null;
try {
Class.forName(DRIVER); // 加载数据库驱动
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD); // 获取数据库连接对象
} catch (ClassNotFoundException e) { // 处理驱动加载异常
e.printStackTrace();
} catch (SQLException e) { // 处理 SQL 异常
e.printStackTrace();
}
return conn;
}
// 关闭数据库连接、PreparedStatement 对象和 ResultSet 对象
public static void close(Connection conn, PreparedStatement ps, ResultSet rs) {
try {
if (rs != null) {
rs.close();
}
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) { // 处理 SQL 异常
e.printStackTrace();
}
}
}
```
在上面的代码中,我们定义了一个常量数组,其中包含了数据库连接信息和 JDBC 驱动信息。在 `getConn()` 方法中,我们首先加载数据库驱动,然后使用 `DriverManager.getConnection()` 方法获取数据库连接对象。在 `close()` 方法中,我们分别关闭 ResultSet 对象、PreparedStatement 对象和 Connection 对象,注意要捕获可能出现的 SQLException 异常。
public CommDAO() { conn = this.getConn(); }
这是一个 Java 代码片段,定义了一个名为 CommDAO 的类,其中包含一个无参构造函数。在该构造函数中,调用了该类中的 getConn() 方法,并将返回的连接对象赋值给成员变量 conn。该构造函数的作用是在实例化 CommDAO 对象时,自动获取数据库连接对象,并将其赋值给 conn 成员变量,以便后续的数据库操作使用该连接对象。需要注意的是,该代码片段缺少类定义和 getConn() 方法的具体实现,无法判断该代码片段的完整性和正确性。
相关推荐
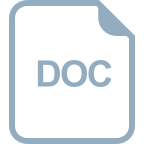
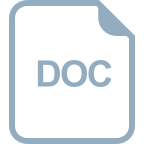
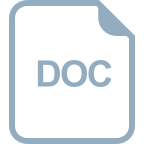













