java中装饰器模式的实现
时间: 2024-05-14 09:17:39 浏览: 8
装饰器模式(Decorator Pattern)是一种结构型设计模式,它允许你动态地将行为添加到对象中,而无需使用继承。装饰器模式是一种包装模式,也就是说,它通过创建一个包装类来包装真正的对象。
在 Java 中,装饰器模式通常通过以下步骤实现:
1. 定义一个抽象的组件类,它是被装饰对象和装饰器对象的共同接口。
```java
public interface Component {
void operation();
}
```
2. 定义具体的组件类,它是被装饰对象的实现。
```java
public class ConcreteComponent implements Component {
@Override
public void operation() {
System.out.println("具体组件的操作");
}
}
```
3. 定义抽象装饰器类,它继承自组件类,并包含一个组件对象的引用。
```java
public abstract class Decorator implements Component {
protected Component component;
public Decorator(Component component) {
this.component = component;
}
@Override
public void operation() {
component.operation();
}
}
```
4. 定义具体的装饰器类,它继承自抽象装饰器类,实现了装饰器所具有的功能。
```java
public class ConcreteDecorator extends Decorator {
public ConcreteDecorator(Component component) {
super(component);
}
@Override
public void operation() {
super.operation();
addedBehavior();
}
private void addedBehavior() {
System.out.println("具体装饰器的操作");
}
}
```
5. 在客户端代码中使用装饰器来包装被装饰对象。
```java
Component component = new ConcreteComponent();
Component decorator = new ConcreteDecorator(component);
decorator.operation();
```
以上就是 Java 中装饰器模式的实现步骤。通过这种方式,我们可以在运行时动态地添加新的行为,而不必对原有的类进行修改。
相关推荐
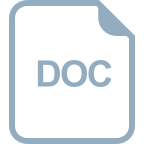














