C#判断字符串为空
时间: 2023-07-12 20:18:50 浏览: 130
在 C# 中,可以使用以下方法来判断字符串是否为空:
1. 使用 String.IsNullOrEmpty() 方法来判断是否为空或 null。
示例代码:
```
string str = "";
if (String.IsNullOrEmpty(str))
{
Console.WriteLine("字符串为空或 null");
}
```
2. 使用 String.IsNullOrWhiteSpace() 方法来判断是否为空、null或者只包含空格。
示例代码:
```
string str = " ";
if (String.IsNullOrWhiteSpace(str))
{
Console.WriteLine("字符串为空或只包含空格");
}
```
建议使用第二种方法,因为它可以同时判断字符串是否为空或者只包含空格。
相关问题
C#如何判断字符串为空
在C#中,判断字符串是否为空可以使用多种方法,以下是常见的几种情况:
1. **空值检查**:
如果变量是`string`类型并且尚未赋值,可以直接使用`null`关键字来判断。
```csharp
string str = null;
if (str == null)
{
Console.WriteLine("String is empty or null");
}
```
2. **长度检查**:
如果你想检查字符串的内容,可以检查其长度是否为0。
```csharp
string str = "";
if (string.IsNullOrEmpty(str) || str.Length == 0)
{
Console.WriteLine("String is empty");
}
```
`string.IsNullOrEmpty()` 方法会同时检查字符串是否为`null`或者长度为0。
3. **等于空字符串**:
直接比较字符串变量是否等于空字符串 ""。
```csharp
if (str == "")
{
Console.WriteLine("String is empty");
}
```
4. **使用条件运算符**:
也可以使用三元运算符 (`? :`) 进行简单的判断。
```csharp
string str = "";
bool isEmpty = str == null || str.Length == 0 ? true : false;
if (isEmpty)
{
Console.WriteLine("String is empty");
}
```
在上述所有情况下,如果字符串确实为空(包括`null`),将会打印出相应的提示信息。
c#判断字符串是否为空
你可以使用 string 类的 IsNullOrEmpty 方法来判断一个字符串是否为空。下面是一个示例:
```csharp
string str = "hello world";
if (string.IsNullOrEmpty(str))
{
Console.WriteLine("字符串为空");
}
else
{
Console.WriteLine("字符串不为空");
}
```
如果字符串为空,那么 IsNullOrEmpty 方法会返回 true。如果不为空,返回 false。
阅读全文
相关推荐
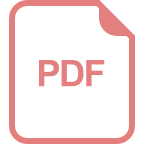



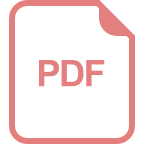
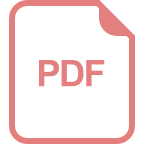
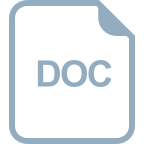




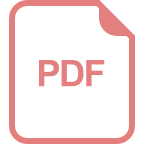
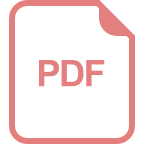