C#判断字符串是否为空
时间: 2024-08-08 19:01:06 浏览: 70
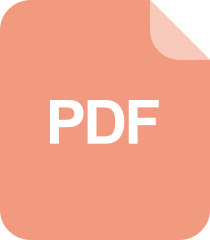
C# 判断字符串第一位是否为数字

在C#中,有几种方法可以判断字符串是否为空:
1. 使用`string.IsNullOrEmpty()` 或 `string.IsNullOrWhiteSpace()` 方法:这两个方法会检查字符串是否为`null`、空字符串或者只包含空白字符。例如:
```csharp
string str = "";
if (string.IsNullOrEmpty(str))
Console.WriteLine("String is null or empty");
```
2. 直接比较:你可以直接使用`==`操作符来检查字符串是否等于空字符串`""`。注意这将不会忽略所有空格或其他空白字符。例如:
```csharp
string str = " ";
if (str == "")
Console.WriteLine("String is empty");
```
3. 使用`string.Length`属性:如果字符串长度为0,那么它就是空的。例如:
```csharp
if (str.Length == 0)
Console.WriteLine("String is empty");
```
阅读全文
相关推荐
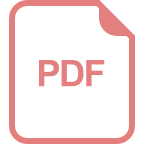
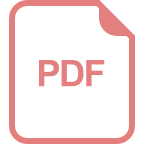


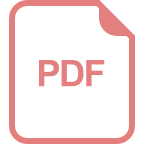
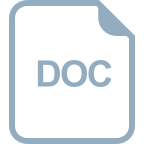



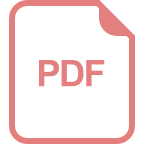
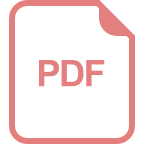





