java 根据pdf url 生成另一个pdf文件 不用PDFBox
时间: 2023-09-30 13:04:43 浏览: 110
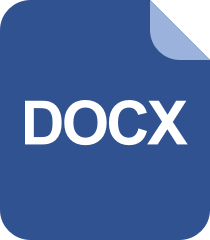
使用java生成pdf文件
可以使用Java中的第三方库iText来实现。以下是一个基本的示例代码:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.URL;
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.pdf.PdfCopy;
import com.itextpdf.text.pdf.PdfReader;
public class PdfGenerator {
public static void main(String[] args) {
String sourceUrl = "http://example.com/source.pdf";
String destinationFile = "destination.pdf";
try {
// 创建一个新的 PDF 文档
Document document = new Document();
PdfCopy copy = new PdfCopy(document, new FileOutputStream(destinationFile));
document.open();
// 从 URL 中读取原始 PDF 文档,逐页复制到新文档中
PdfReader reader = new PdfReader(new URL(sourceUrl));
int n = reader.getNumberOfPages();
for (int i = 0; i < n;) {
copy.addPage(copy.getImportedPage(reader, ++i));
}
// 关闭文档
document.close();
reader.close();
} catch (DocumentException | IOException e) {
e.printStackTrace();
}
}
}
```
这个示例使用iText的PdfCopy类从指定的PDF URL中读取原始文档并复制到新生成的PDF文件中。注意,在使用这个代码之前,你需要下载和导入iText库,可以在 Maven 中添加以下依赖项:
```xml
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13.2</version>
</dependency>
```
阅读全文
相关推荐

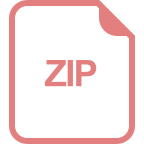
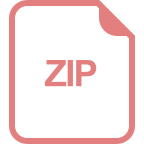
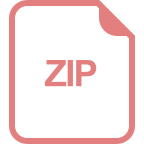
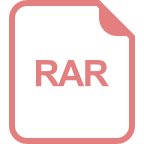
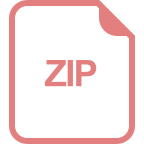
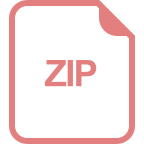
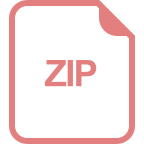
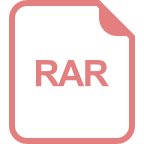
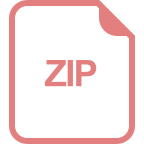
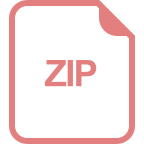
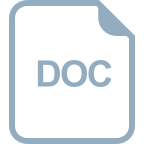
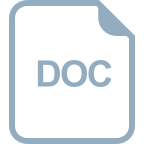
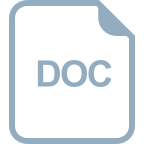
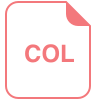
