用C语言写一个链队列实现的银行取钱模拟
时间: 2023-04-06 13:04:42 浏览: 68
好的,我可以回答这个问题。以下是用C语言写一个链队列实现的银行取钱模拟的代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
typedef struct queue {
Node *front;
Node *rear;
} Queue;
void initQueue(Queue *q) {
q->front = q->rear = (Node *)malloc(sizeof(Node));
q->front->next = NULL;
}
int isEmpty(Queue *q) {
return q->front == q->rear;
}
void enQueue(Queue *q, int data) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
q->rear->next = newNode;
q->rear = newNode;
}
int deQueue(Queue *q) {
if (isEmpty(q)) {
printf("Queue is empty.\n");
return -1;
}
Node *p = q->front->next;
int data = p->data;
q->front->next = p->next;
if (q->rear == p) {
q->rear = q->front;
}
free(p);
return data;
}
int main() {
Queue q;
initQueue(&q);
int choice, amount;
do {
printf("1. Deposit\n");
printf("2. Withdraw\n");
printf("3. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter amount to deposit: ");
scanf("%d", &amount);
enQueue(&q, amount);
printf("Deposit successful.\n");
break;
case 2:
printf("Enter amount to withdraw: ");
scanf("%d", &amount);
if (amount > deQueue(&q)) {
printf("Withdrawal failed. Insufficient balance.\n");
} else {
printf("Withdrawal successful.\n");
}
break;
case 3:
printf("Exiting...\n");
break;
default:
printf("Invalid choice.\n");
}
} while (choice != 3);
return 0;
}
```
这个程序实现了一个银行取钱模拟,使用了链队列来存储用户的存款和取款请求。用户可以选择存款、取款或退出程序。如果用户选择存款,程序会要求输入存款金额,并将其加入队列中;如果用户选择取款,程序会要求输入取款金额,并从队列中取出相应的金额进行比较,如果余额不足则取款失败,否则取款成功。如果用户选择退出程序,程序会结束运行。
相关推荐
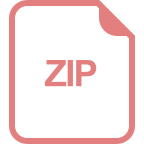














