用C#语言wpf窗体完成下题在窗体中显示字符,每隔1秒字符移动一定距离,先右移,移到右边界,再左移,移到左边界,又一次右移,如此循环。(提示:修改Label 的Margin属性)
时间: 2024-11-15 09:26:03 浏览: 2
在C# WPF中,你可以创建一个简单的窗体应用来实现这个效果。首先,你需要一个`Label`控件来显示字符,并且需要处理时间间隔和位置更新。下面是一个基本步骤:
1. 创建一个新的WPF项目并打开主窗口.xaml文件。
```xml
<Window x:Class="YourNamespace.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Character Movement" Height="450" Width="800">
<StackPanel>
<Label Name="MovingLabel" Content="A" Margin="0,50,0,0"/>
</StackPanel>
</Window>
```
2. 在`.cs`文件中,添加一个公共方法用于处理动画和计时器:
```csharp
using System;
using System.Windows.Threading;
public partial class MainWindow : Window
{
private int moveDistance = 100; // 字符每次移动的距离
private DispatcherTimer timer; // 计时器
public MainWindow()
{
InitializeComponent();
// 初始化计时器
timer = new DispatcherTimer();
timer.Interval = TimeSpan.FromSeconds(1);
timer.Tick += Timer_Tick;
timer.Start();
}
private void Timer_Tick(object sender, EventArgs e)
{
// 更新标签的位置
int leftBorder = this.Left + this.Width - MovingLabel.ActualWidth;
int rightBorder = this.Left;
int direction = MovingLabel.Margin.Left > leftBorder ? -moveDistance : moveDistance;
double newX = Math.Max(rightBorder, Math.Min(leftBorder, MovingLabel.Margin.Left + direction));
if (newX == leftBorder) // 到达左侧边界开始向右移动
direction = moveDistance;
MovingLabel.Margin = new Thickness(newX, MovingLabel.Margin.Top, 0, MovingLabel.Margin.Bottom);
}
}
```
3. 运行程序,你会看到一个字符在窗体中按照指定规则来回移动。
阅读全文
相关推荐
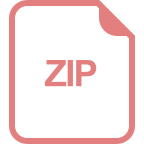
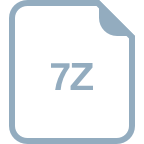
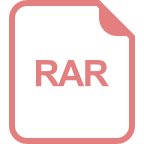
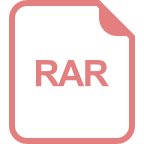



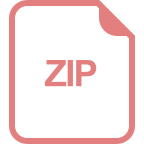
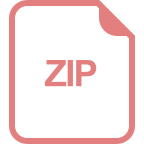
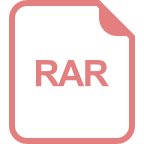
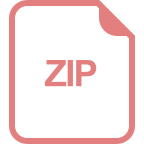
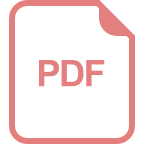
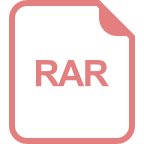
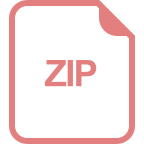
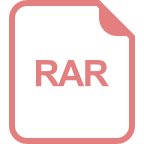
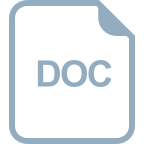

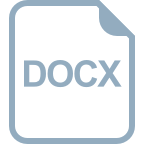
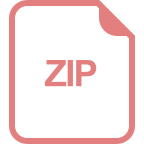