用Java编写一个应用程序,实现如下图所示的图形界面,要求单击按钮将窗口的背景颜色设置为相应的颜色。设置颜色可以调用容器的setBackground(Color c)方法,参数Color可以使用java awt.Color类的常量,如Color.RED等。
时间: 2024-02-19 12:59:29 浏览: 113
好的,以下是用Java Swing编写的代码,实现上述功能:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ColorSetter extends JFrame implements ActionListener {
private JButton redButton;
private JButton greenButton;
private JButton blueButton;
public ColorSetter() {
// 设置窗口标题
setTitle("Color Setter");
// 创建按钮
redButton = new JButton("Red");
greenButton = new JButton("Green");
blueButton = new JButton("Blue");
// 添加按钮的监听器
redButton.addActionListener(this);
greenButton.addActionListener(this);
blueButton.addActionListener(this);
// 添加控件到窗口
Container contentPane = getContentPane();
contentPane.setLayout(new FlowLayout());
contentPane.add(redButton);
contentPane.add(greenButton);
contentPane.add(blueButton);
// 设置窗口大小和位置
setSize(300, 100);
setLocationRelativeTo(null);
}
// 按钮的监听器实现
public void actionPerformed(ActionEvent e) {
if (e.getSource() == redButton) {
getContentPane().setBackground(Color.RED);
} else if (e.getSource() == greenButton) {
getContentPane().setBackground(Color.GREEN);
} else if (e.getSource() == blueButton) {
getContentPane().setBackground(Color.BLUE);
}
}
public static void main(String[] args) {
ColorSetter frame = new ColorSetter();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
```
在这个程序中,我们创建了一个继承自JFrame的窗口,包括三个按钮。当单击按钮时,按钮的监听器会将窗口的背景颜色设置为相应的颜色。我们把这个程序保存为ColorSetter.java,并编译运行即可。
阅读全文
相关推荐









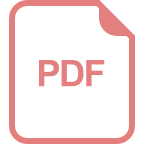
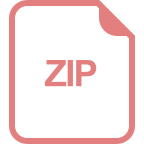
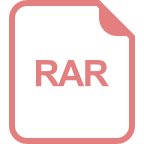
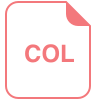

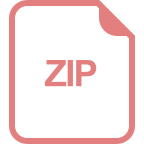
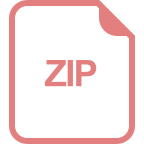