espnow esp32 远程控制 led
时间: 2024-02-15 09:33:24 浏览: 113
以下是使用ESP-NOW协议远程控制ESP32板子上LED的步骤:
1. 在ESP32板子上,创建一个新的Arduino IDE项目。
2. 在程序中导入ESP-NOW库:
```
#include <esp_now.h>
```
3. 定义一个结构体来保存ESP-NOW发送数据的MAC地址和数据:
```
typedef struct __attribute__((packed)) {
uint8_t mac[6];
uint8_t data;
} __packed message_t;
```
4. 在setup()函数中初始化ESP-NOW库:
```
WiFi.mode(WIFI_STA); // 设置为STA模式
if (esp_now_init() != ESP_OK) { // 初始化ESP-NOW
Serial.println("Error initializing ESP-NOW");
return;
}
```
5. 在setup()函数中设置要发送数据的MAC地址:
```
esp_now_peer_info_t peer;
memcpy(peer.peer_addr, MAC_ADDRESS, 6); // 设置MAC地址
peer.channel = 0; // 设置通道
peer.encrypt = false; // 不加密
if (esp_now_add_peer(&peer) != ESP_OK) { // 添加对等节点
Serial.println("Failed to add peer");
return;
}
```
6. 在loop()函数中检测是否有接收到消息:
```
message_t receivedMessage;
int packetSize = sizeof(receivedMessage);
if (esp_now_recv(NULL, &receivedMessage, &packetSize) == ESP_OK) { // 接收数据成功
Serial.print("Received message: ");
Serial.println(receivedMessage.data);
digitalWrite(LED_PIN, receivedMessage.data); // 控制LED
}
```
7. 在loop()函数中定期发送数据:
```
message_t sendMessage;
memcpy(sendMessage.mac, MAC_ADDRESS, 6); // 设置MAC地址
sendMessage.data = digitalRead(BUTTON_PIN); // 设置数据为按钮状态
esp_err_t result = esp_now_send(NULL, &sendMessage, sizeof(sendMessage)); // 发送数据
if (result == ESP_OK) {
Serial.println("Sent message successfully");
} else if (result == ESP_ERR_ESPNOW_NOT_INIT) {
Serial.println("ESP-NOW not initialized");
} else if (result == ESP_ERR_ESPNOW_ARG) {
Serial.println("Invalid argument");
} else if (result == ESP_ERR_ESPNOW_INTERNAL) {
Serial.println("Internal error");
} else if (result == ESP_ERR_ESPNOW_NO_MEM) {
Serial.println("Out of memory");
} else if (result == ESP_ERR_ESPNOW_NOT_FOUND) {
Serial.println("Peer not found");
} else {
Serial.println("Unknown error");
}
```
8. 在setup()函数中设置LED和按钮的GPIO:
```
pinMode(LED_PIN, OUTPUT);
pinMode(BUTTON_PIN, INPUT_PULLUP);
```
9. 在程序中定义MAC_ADDRESS常量,该常量用于设置要发送数据的MAC地址:
```
#define MAC_ADDRESS {0x12, 0x34, 0x56, 0x78, 0x9A, 0xBC} // 设置MAC地址
```
注意:需要将MAC_ADDRESS替换为实际的MAC地址。可以使用ESP32板子上的“ESP-NOW Example”示例程序来获取MAC地址。
完成以上步骤后,可以将程序上传到ESP32板子上,并使用另一个ESP32板子或其他支持ESP-NOW协议的设备来远程控制LED。
阅读全文
相关推荐
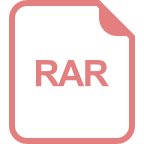















