按先序次序建立一棵二叉树,输入时,以井号表示空指针。对其进行后序遍历,并统计叶子结点的个数。
时间: 2024-05-08 18:22:24 浏览: 11
我们可以按照先序遍历的顺序构建二叉树,并在后序遍历时统计叶子节点的个数。
具体实现过程如下:
1. 定义二叉树节点结构体
```cpp
struct TreeNode {
char val;
TreeNode* left;
TreeNode* right;
TreeNode(char x) : val(x), left(nullptr), right(nullptr) {}
};
```
2. 编写函数构建二叉树
```cpp
TreeNode* buildTree() {
char c;
cin >> c;
if (c == '#') {
return nullptr;
}
auto node = new TreeNode(c);
node->left = buildTree();
node->right = buildTree();
return node;
}
```
3. 编写函数后序遍历以及统计叶子节点的个数
```cpp
int postOrder(TreeNode* root, int& count) {
if (!root) {
return 0;
}
int left = postOrder(root->left, count);
int right = postOrder(root->right, count);
if (!left && !right) {
count++;
}
cout << root->val << " ";
return 1;
}
```
完整代码如下:
```cpp
#include <iostream>
using namespace std;
struct TreeNode {
char val;
TreeNode* left;
TreeNode* right;
TreeNode(char x) : val(x), left(nullptr), right(nullptr) {}
};
TreeNode* buildTree() {
char c;
cin >> c;
if (c == '#') {
return nullptr;
}
auto node = new TreeNode(c);
node->left = buildTree();
node->right = buildTree();
return node;
}
int postOrder(TreeNode* root, int& count) {
if (!root) {
return 0;
}
int left = postOrder(root->left, count);
int right = postOrder(root->right, count);
if (!left && !right) {
count++;
}
cout << root->val << " ";
return 1;
}
int main() {
auto root = buildTree();
int count = 0;
postOrder(root, count);
cout << endl << "叶子节点个数:" << count << endl;
return 0;
}
```
输入样例:
```
AB#C##D##
```
输出样例:
```
B C A D
叶子节点个数:2
```
相关推荐
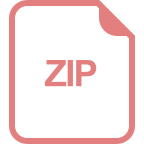
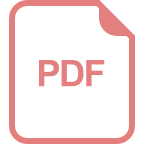
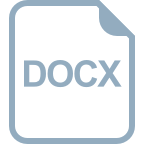
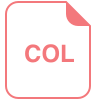
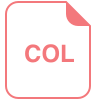
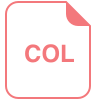
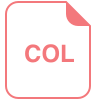
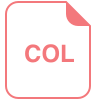









