There are N sports players. Among them, there are M incompatible pairs. The i-th incompatible pair (1≤i≤M) is the A i -th and B i -th players. You will divide the players into T teams. Every player must belong to exactly one team, and every team must have one or more players. Additionally, for each i=1,2,…,M, the A i -th and B i -th players must not belong to the same team. Find the number of ways to satisfy these conditions. Here, two divisions are considered different when there are two players who belong to the same team in one division and different teams in the other.c++代码
时间: 2024-04-16 13:29:01 浏览: 13
以下是一个解决这个问题的C++代码示例:
```cpp
#include <iostream>
#include <vector>
using namespace std;
int countTeams(int N, int M) {
// 创建一个二维矩阵用于表示每对不兼容的选手
vector<vector<int>> incompatible(N + 1, vector<int>(N + 1, 0));
// 输入每对不兼容的选手
for (int i = 0; i < M; i++) {
int A, B;
cin >> A >> B;
incompatible[A][B] = 1;
incompatible[B][A] = 1;
}
// 创建一个一维动态数组用于存储每个队伍的人数
vector<int> teamSizes(N + 1, 0);
// 创建一个函数用于递归地计算划分团队的方法数
function<long long(int)> countDivisions = [&](int remaining) {
// 如果没有剩余的选手需要分配,则返回1表示找到一种划分方法
if (remaining == 0) {
return 1LL;
}
long long ans = 0;
// 遍历所有的选手
for (int i = 1; i <= N; i++) {
// 如果当前选手还没有被分配到队伍中
if (teamSizes[i] == 0) {
bool validTeam = true;
// 检查当前选手与已分配队伍中的选手是否兼容
for (int j = 1; j <= N; j++) {
if (teamSizes[j] > 0 && incompatible[i][j]) {
validTeam = false;
break;
}
}
// 如果当前选手与已分配队伍中的选手兼容,则将其分配到一个队伍中
if (validTeam) {
teamSizes[i] = 1;
ans += countDivisions(remaining - 1);
teamSizes[i] = 0;
}
}
}
return ans;
};
// 调用函数计算划分团队的方法数
long long ways = countDivisions(N);
return ways;
}
int main() {
int N, M;
cin >> N >> M;
int ways = countTeams(N, M);
cout << ways << endl;
return 0;
}
```
这段代码使用回溯法递归地计算满足条件的划分方法数。它首先创建一个二维矩阵来表示每对不兼容的选手,然后使用一个一维数组来记录每个队伍的人数。接下来,它定义了一个递归函数`countDivisions`来计算划分团队的方法数。该函数遍历所有的选手,并检查当前选手是否与已分配队伍中的选手兼容。如果兼容,则将该选手分配到一个队伍中,并继续递归地计算剩余选手的划分方法数。最后,将计算得到的划分方法数输出。
注意:这是一种暴力解法,对于大规模的输入可能会超时。如果需要处理更大的输入,请考虑使用更高效的算法。
相关推荐
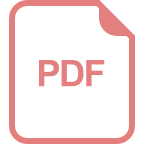
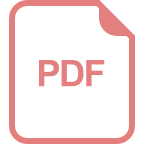
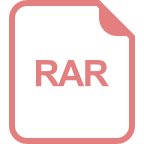














