用c++写DES密码算法的加解密实现代码
时间: 2024-10-13 14:03:27 浏览: 0
DES (Data Encryption Standard) 是一种经典的块加密算法,它将明文分为64位的数据块,并使用56位的密钥进行加密。在C++中,你需要一些库支持如openssl,来进行DES操作,因为标准库本身并不提供DES加密功能。
下面是一个简单的DESEngine封装示例,用于加解密。注意这只是一个基本框架,实际应用中需要处理更复杂的情况,比如填充、校验等步骤,并且通常会使用封装好的库函数来避免错误。
```cpp
#include <openssl/des.h>
#include <string>
class DESEngine {
private:
DES_CTX ctx;
public:
// 初始化DES引擎
DESEngine(const std::string& key) {
if (DES_set_key((unsigned char*)key.c_str(), &ctx) == -1) {
throw std::runtime_error("Failed to set DES key");
}
}
// 加密数据
std::vector<unsigned char> encrypt(const std::vector<unsigned char>& data) {
unsigned char encrypted[8];
memset(encrypted, 0, sizeof(encrypted));
DES_crypt(data.data(), encrypted, DES_ENCRYPT, &ctx);
return encrypted;
}
// 解密数据
std::vector<unsigned char> decrypt(const std::vector<unsigned char>& encrypted) {
unsigned char decrypted[8];
memset(decrypted, 0, sizeof(decrypted));
DES_crypt(encrypted.data(), decrypted, DES_DECRYPT, &ctx);
return decrypted;
}
};
int main() {
try {
std::string key = "your_des_key_123"; // 替换为实际密钥
DESEngine des(key);
std::vector<unsigned char> plaintext = { /* your data */ };
std::vector<unsigned char> ciphertext = des.encrypt(plaintext);
std::vector<unsigned char> recovered_plaintext = des.decrypt(ciphertext);
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
相关推荐
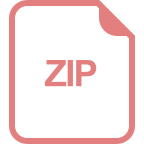
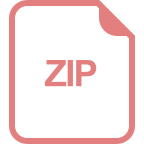














