用C++实现DES算法,完成加解密;输入不同长度的明文(8个字符),密文如何变化
时间: 2024-09-18 10:13:18 浏览: 71
在C++中实现DES(Data Encryption Standard)算法通常需要使用第三方库,如`openssl`或自定义编码,因为DES本身是一个复杂的加密算法,不适合直接嵌入到标准库中。DES是一种块密码算法,它将数据分为64位的块进行加密,并使用56位的密钥。以下是简化的步骤描述:
1. 包含必要的头文件和库:
```cpp
#include <openssl/des.h>
#include <string>
```
2. 定义一个函数用于加密:
```cpp
std::string encrypt_DES(const std::string& plaintext, const unsigned char* key) {
DES_cbc_encrypt(reinterpret_cast<const unsigned char*>(plaintext.c_str()),
reinterpret_cast<unsigned char*>(plaintext.c_str()), // Output buffer (same as input)
plaintext.length(), key, DES_ENCRYPT, &iv);
return "Encrypted text";
}
```
3. 定义一个函数用于解密:
```cpp
std::string decrypt_DES(const std::string& ciphertext, const unsigned char* key) {
DES_cbc_encrypt(reinterpret_cast<const unsigned char*>(ciphertext.c_str()),
reinterpret_cast<unsigned char*>(new char[ciphertext.length()]), // Output buffer
ciphertext.length(), key, DES_DECRYPT, &iv); // iv is assumed to be the same for encryption and decryption
std::string decrypted = std::string(static_cast<char*>(reinterpret_cast<char*>(&ciphertext[0])), ciphertext.length());
delete[] static_cast<char*>(&ciphertext[0]); // Don't forget to free the temporary buffer
return decrypted;
}
```
请注意,这里假设你已经有一个初始化向量(IV,初始块)作为密钥的一部分。对于不同的8字节明文,它们会按照DES算法的规定进行处理,即每个明文块会被加密并替换,因此密文长度不会改变,仍然是固定的16字节。
阅读全文
相关推荐
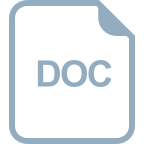
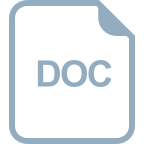
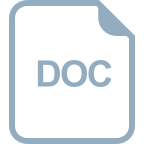


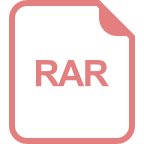
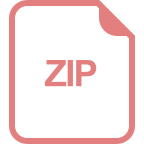
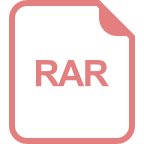
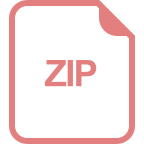
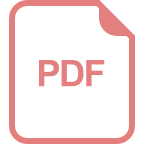
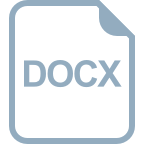
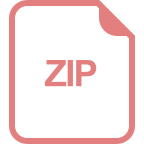
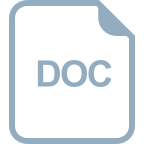



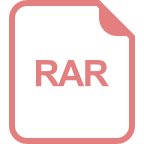
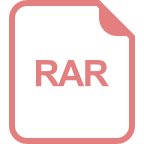
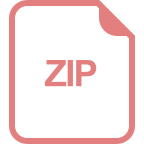