用C++或python实现DES算法,完成加解密;输入不同长度的明文(8个字符),密文如何变化;
时间: 2024-09-24 16:17:00 浏览: 71
DES (Data Encryption Standard) 算法是一种对称加密技术,用于保护数据的安全传输。在C++和Python中实现DES通常涉及到以下几个步骤:
**在C++中实现DES(使用Crypto++库):**
```cpp
#include <cryptopp/des.h>
#include <iostream>
std::string des_encrypt(const std::string &plaintext, const std::string &key) {
CryptoPP::DES::Encryption encryption(key, CryptoPP::DEFAULT_KEYLENGTH);
std::string ciphertext((const char*)encryption.ProcessMessage((const unsigned char*)plaintext.c_str()), plaintext.length());
return ciphertext;
}
std::string des_decrypt(const std::string &ciphertext, const std::string &key) {
CryptoPP::DES::Decryption decryption(key, CryptoPP::DEFAULT_KEYLENGTH);
std::string decrypted((char*)decryption.ProcessMessage((const unsigned char*)ciphertext.c_str()), ciphertext.length());
return decrypted;
}
int main() {
std::string key = "your_key_16_chars"; // 8字节的密钥
std::string plaintext("abcdefgh"); // 8字节的明文
std::string encrypted = des_encrypt(plaintext, key);
std::cout << "Encrypted: " << encrypted << std::endl;
std::string decrypted = des_decrypt(encrypted, key);
if (decrypted == plaintext)
std::cout << "Decrypted successfully." << std::endl;
else
std::cout << "Decryption failed." << std::endl;
return 0;
}
```
**在Python中实现DES(使用pyDes库):**
```python
from pyDes import DES, ECB
def des_encrypt(plaintext, key):
k = DES(key, mode=ECB, pad=None, padmode=PAD_PKCS5)
ciphertext = k.encrypt(plaintext.encode('utf-8'))
return ciphertext.decode('utf-8')
def des_decrypt(ciphertext, key):
k = DES(key, mode=ECB, pad=None, padmode=PAD_PKCS5)
decrypted = k.decrypt(ciphertext.encode('utf-8')).decode('utf-8')
return decrypted
plaintext = "abcdefgh"
key = b"your_key_16_bytes" # 8字节的密钥
encrypted = des_encrypt(plaintext, key)
print(f"Encrypted: {encrypted}")
decrypted = des_decrypt(encrypted, key)
if decrypted == plaintext:
print("Decrypted successfully.")
else:
print("Decryption failed.")
```
当你使用DES加密8字节的明文时,由于DES是对64位的数据块操作的,超过这个长度的部分会被切成64位的块进行加密。例如,如果明文是`abcdefghijk`,它会被分块成`abcdef`和`ghijk`。每次加密都会得到一个新的64位密文块,因此密文长度可能会比明文略长。
**相关问题--:**
1. C++和Python实现DES有何区别?
2. DES加密后如何验证数据完整性?
3. DES的安全性和现代加密标准相比如何?
阅读全文
相关推荐
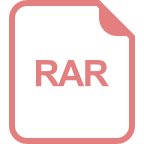
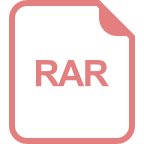
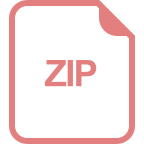















