用C++或python实现DES算法,完成加解密; 输入不同长度的明文(8个字符),密文如何变化;
时间: 2024-09-25 13:02:16 浏览: 81
在C++和Python中,DES(Data Encryption Standard)算法通常需要第三方库支持,因为其涉及到复杂的位操作和密钥管理。以下是简化的示例,展示了如何使用`openssl`库在C++和`pycryptodome`库在Python中实现DES的加密和解密:
**C++ (使用OpenSSL库)**
```cpp
#include <openssl/des.h>
#include <string>
std::string DES_encrypt(const std::string& plaintext, const unsigned char* key) {
DES_cbc_encrypt(reinterpret_cast<const unsigned char*>(plaintext.c_str()),
reinterpret_cast<unsigned char*>(plaintext.c_str()), // 输出将覆盖输入
plaintext.length(), key, DES_ENCRYPT, &iv);
return std::string(reinterpret_cast<char*>(plaintext.c_str()));
}
std::string DES_decrypt(const std::string& ciphertext, const unsigned char* key) {
DES_cbc_encrypt(reinterpret_cast<const unsigned char*>(ciphertext.c_str()),
reinterpret_cast<unsigned char*>(ciphertext.c_str()), // 输出将覆盖输入
ciphertext.length(), key, DES_DECRYPT, &iv);
return std::string(reinterpret_cast<char*>(ciphertext.c_str()));
}
```
这里假设`iv`是一个预先设定的初始化向量。注意,DES的块大小固定为64位,所以如果输入的明文不是64位的倍数,边缘部分会被填充。
**Python (使用PyCryptodome库)**
```python
from Crypto.Cipher import DES
from Crypto.Random import get_random_bytes
def DES_encrypt(plaintext, key):
cipher = DES.new(key, DES.MODE_CBC)
padded_plaintext = plaintext + b'\0' * (8 - len(plaintext) % 8)
encrypted = cipher.encrypt(padded_plaintext)
return encrypted
def DES_decrypt(ciphertext, key):
cipher = DES.new(key, DES.MODE_CBC)
decrypted = cipher.decrypt(ciphertext)
# Remove padding from the last byte, which should be a number between 1 and 8
unpadded_decrypted = decrypted[:-decrypted[-1]]
return unpadded_decrypted.decode()
# 示例用法
key = get_random_bytes(8) # Generate a random 8-byte key
plaintext = "Test" * 8 # 8-character string (will be padded to 64 bits)
encrypted_text = DES_encrypt(plaintext.encode(), key)
print("Encrypted:", encrypted_text)
decrypted_text = DES_decrypt(encrypted_text, key)
print("Decrypted:", decrypted_text)
```
对于不同长度的明文,例如8个字符,会先进行填充使其达到64位(8字节)。加密后的密文长度会因为填充而变长。由于DES本身的限制,它并不直接处理非块大小的数据,因此不会按字符边界改变,而是整体加密或解密。
阅读全文
相关推荐
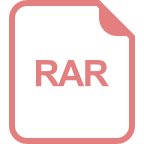
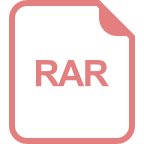
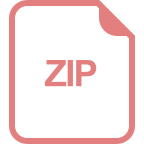

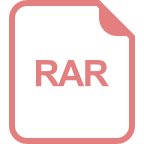
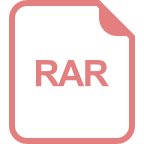
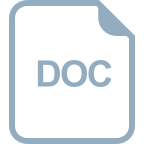
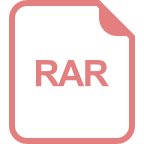
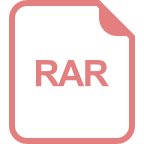
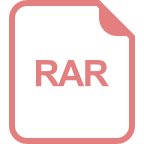
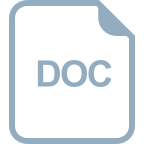
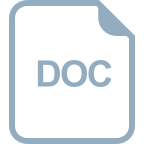
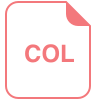
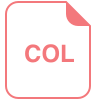
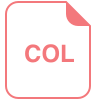
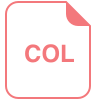
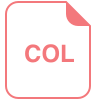
