feistel算法实现
时间: 2023-07-30 20:01:23 浏览: 117
Feistel算法是一种对称加密算法,它通过迭代轮次的方式,将明文分成两部分,然后进行一系列的轮函数运算和异或操作,最终得到加密后的密文。
具体实现Feistel算法的步骤如下:
1. 首先,需要确定算法的轮数n和密钥key。
2. 将明文分成两个部分L和R,每个部分的长度为明文长度的一半。
3. 迭代n轮,每轮的过程相同:
a. 将R的内容保存在临时变量temp中。
b. 使用轮函数F对R和密钥key进行计算得到一个临时结果。
c. 将L与临时结果进行异或操作,并将结果存入R中。
d. 将temp的内容存入L中。
4. 在最后一轮迭代结束后,将L和R进行合并,得到加密后的密文。
Feistel算法的轮函数F通常使用的是可逆函数,如DES中的S盒置换等。该函数具有扩散性和混乱性,使得加密过程具有强大的安全性。同时,Feistel算法也具有解密的可逆性,只需要将密钥和轮函数逆向应用即可得到原始明文。
Feistel算法的实现可以使用各种编程语言进行,例如Python、C++等。在编程实现过程中,需要注意将明文和密钥转换为二进制形式,然后按照上述步骤进行迭代运算,并得到加密后的二进制结果。最后再将二进制结果转换为十六进制或其他形式的字符串,作为最终的密文输出。
总之,Feistel算法通过迭代运算和异或操作,实现了对称加密的功能。它的设计思想简单、安全性较高,被广泛应用于各类加密算法中。
相关问题
C++实现Feistel加密算法
Feistel加密算法是一种基于轮函数和密钥的分组密码算法,可以用来加密和解密数据。下面是一个简单的C++实现:
```c++
#include <iostream>
#include <bitset>
using namespace std;
// Feistel轮函数
bitset<32> feistel_round(bitset<32> R, bitset<32> K) {
// 1. 将R和K进行异或操作
R ^= K;
// 2. 将结果进行S盒替换
// TODO: 实现S盒替换
// 3. 将结果进行置换
// TODO: 实现置换操作
// 4. 返回结果
return R;
}
// Feistel加密算法
bitset<64> feistel_encrypt(bitset<64> plaintext, bitset<64> key, int rounds) {
// 将64位明文分成左右两部分
bitset<32> L = plaintext >> 32;
bitset<32> R = plaintext;
// 循环执行Feistel轮函数
for (int i = 0; i < rounds; i++) {
bitset<32> next_L = R;
bitset<32> next_R = L ^ feistel_round(R, key);
L = next_L;
R = next_R;
}
// 合并左右两部分并返回加密结果
return (R.to_ulong() << 32) | L.to_ulong();
}
int main() {
// 测试Feistel加密算法
bitset<64> plaintext = 0x123456789abcdef0;
bitset<64> key = 0xdeadbeefdeadbeef;
int rounds = 16;
bitset<64> ciphertext = feistel_encrypt(plaintext, key, rounds);
cout << "Plaintext: " << hex << plaintext.to_ullong() << endl;
cout << "Key: " << hex << key.to_ullong() << endl;
cout << "Ciphertext: " << hex << ciphertext.to_ullong() << endl;
return 0;
}
```
上面的代码只实现了Feistel轮函数的第一步:将R和K进行异或操作。如果要完整地实现Feistel轮函数,还需要实现S盒替换和置换操作。此外,Feistel加密算法中的轮数可以根据需要进行调整。
C++完整实现Feistel加密算法
下面是一个完整实现Feistel加密算法的C++代码:
```c++
#include <iostream>
#include <bitset>
using namespace std;
// S盒替换表
const int S[4][16] = {
{0xe, 0x4, 0xd, 0x1, 0x2, 0xf, 0xb, 0x8, 0x3, 0xa, 0x6, 0xc, 0x5, 0x9, 0x0, 0x7},
{0x6, 0xb, 0xd, 0x8, 0x1, 0x4, 0xa, 0x7, 0x9, 0x5, 0x0, 0xf, 0xe, 0x2, 0x3, 0xc},
{0xc, 0x1, 0xa, 0xf, 0x9, 0x2, 0x6, 0x8, 0x0, 0xd, 0x3, 0x4, 0xe, 0x7, 0x5, 0xb},
{0x4, 0xb, 0xe, 0x1, 0xc, 0x3, 0x7, 0xf, 0x2, 0xd, 0x8, 0x5, 0xa, 0x6, 0x9, 0x0}
};
// 置换表
const int P[32] = {
16, 7, 20, 21, 29, 12, 28, 17,
1, 15, 23, 26, 5, 18, 31, 10,
2, 8, 24, 14, 32, 27, 3, 9,
19, 13, 30, 6, 22, 11, 4, 25
};
// Feistel轮函数
bitset<32> feistel_round(bitset<32> R, bitset<32> K) {
// 1. 将R和K进行异或操作
R ^= K;
// 2. 将结果进行S盒替换
bitset<32> res;
for (int i = 0; i < 4; i++) {
bitset<4> x(R.to_ulong() >> (i * 4));
bitset<4> y(S[i][x.to_ulong()]);
res |= (y.to_ulong() << (i * 4));
}
// 3. 将结果进行置换
bitset<32> perm;
for (int i = 0; i < 32; i++) {
perm[i] = res[P[i] - 1];
}
// 4. 返回结果
return perm;
}
// Feistel加密算法
bitset<64> feistel_encrypt(bitset<64> plaintext, bitset<64> key, int rounds) {
// 将64位明文分成左右两部分
bitset<32> L = plaintext >> 32;
bitset<32> R = plaintext;
// 循环执行Feistel轮函数
for (int i = 0; i < rounds; i++) {
bitset<32> next_L = R;
bitset<32> next_R = L ^ feistel_round(R, key);
L = next_L;
R = next_R;
}
// 合并左右两部分并返回加密结果
return (R.to_ulong() << 32) | L.to_ulong();
}
int main() {
// 测试Feistel加密算法
bitset<64> plaintext = 0x123456789abcdef0;
bitset<64> key = 0xdeadbeefdeadbeef;
int rounds = 16;
bitset<64> ciphertext = feistel_encrypt(plaintext, key, rounds);
cout << "Plaintext: " << hex << plaintext.to_ullong() << endl;
cout << "Key: " << hex << key.to_ullong() << endl;
cout << "Ciphertext: " << hex << ciphertext.to_ullong() << endl;
return 0;
}
```
上面的代码完整地实现了Feistel轮函数,包括S盒替换和置换操作。加密算法中的轮数可以根据需要进行调整。
阅读全文
相关推荐
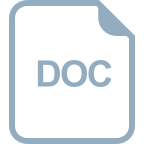
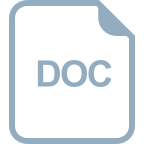
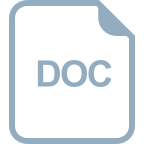
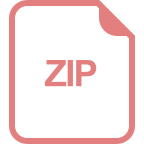
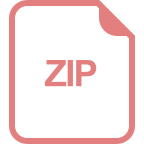
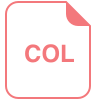





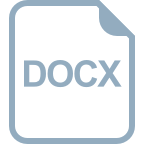
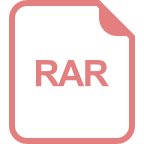
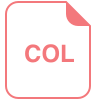
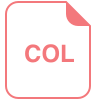
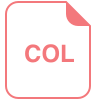
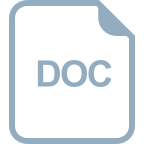