c实现feistel结构加密
时间: 2024-01-17 15:02:47 浏览: 38
Feistel结构是一种对称加密算法的设计思想,主要思想是将明文分成左右两部分,然后交替进行加密和解密,直到达到预定的轮数。最常见的Feistel结构加密算法是DES算法。
下面是一个简单的C语言实现Feistel结构加密的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BLOCK_SIZE 8 // 块大小(字节数)
#define ROUNDS 16 // 加密轮数
#define KEY_SIZE 8 // 密钥大小(字节数)
// S盒
const unsigned char S_BOX[4][16] = {
{0x0E, 0x04, 0x0D, 0x01, 0x02, 0x0F, 0x0B, 0x08, 0x03, 0x0A, 0x06, 0x0C, 0x05, 0x09, 0x00, 0x07},
{0x00, 0x01, 0x07, 0x0D, 0x0F, 0x06, 0x0C, 0x0B, 0x05, 0x08, 0x04, 0x02, 0x09, 0x0E, 0x03, 0x0A},
{0x0B, 0x03, 0x05, 0x08, 0x02, 0x0F, 0x0A, 0x0D, 0x0E, 0x01, 0x07, 0x04, 0x0C, 0x09, 0x00, 0x06},
{0x07, 0x0E, 0x0C, 0x0B, 0x0D, 0x01, 0x03, 0x09, 0x00, 0x02, 0x04, 0x0A, 0x0F, 0x05, 0x08, 0x06}
};
// P盒
const unsigned char P_BOX[BLOCK_SIZE] = {2, 6, 3, 1, 4, 8, 5, 7};
// 左右分块
void split(unsigned char* block, unsigned char* left, unsigned char* right) {
memcpy(left, block, BLOCK_SIZE / 2);
memcpy(right, block + BLOCK_SIZE / 2, BLOCK_SIZE / 2);
}
// 合并左右块
void merge(unsigned char* block, unsigned char* left, unsigned char* right) {
memcpy(block, left, BLOCK_SIZE / 2);
memcpy(block + BLOCK_SIZE / 2, right, BLOCK_SIZE / 2);
}
// F函数
void F(unsigned char* right, unsigned char* subkey) {
unsigned char temp[BLOCK_SIZE / 2];
memcpy(temp, right, BLOCK_SIZE / 2);
memset(right, 0, BLOCK_SIZE / 2);
// 扩展置换
unsigned char expand[BLOCK_SIZE] = {4, 1, 2, 3, 2, 3, 4, 1};
unsigned char expanded[BLOCK_SIZE];
for (int i = 0; i < BLOCK_SIZE; i++) {
expanded[i] = temp[expand[i] - 1];
}
// 异或子密钥
for (int i = 0; i < BLOCK_SIZE / 2; i++) {
expanded[i] ^= subkey[i];
}
// S盒替换
unsigned char row = (expanded[0] << 1) + expanded[3];
unsigned char col = (expanded[1] << 3) + (expanded[2] << 2) + (expanded[4] << 1) + expanded[5];
unsigned char sbox_out = S_BOX[row][col];
for (int i = 0; i < 4; i++) {
right[i] = (sbox_out >> (3 - i)) & 1;
}
// 置换
unsigned char permuted[BLOCK_SIZE / 2];
for (int i = 0; i < BLOCK_SIZE / 2; i++) {
permuted[i] = right[P_BOX[i] - 1];
}
memcpy(right, permuted, BLOCK_SIZE / 2);
}
// Feistel加密
void feistel_encrypt(unsigned char* block, unsigned char* key) {
unsigned char left[BLOCK_SIZE / 2], right[BLOCK_SIZE / 2];
split(block, left, right);
for (int i = 0; i < ROUNDS; i++) {
F(right, key + i * (BLOCK_SIZE / 2));
// 异或左右块
for (int j = 0; j < BLOCK_SIZE / 2; j++) {
left[j] ^= right[j];
}
// 交换左右块
if (i != ROUNDS - 1) {
unsigned char temp[BLOCK_SIZE / 2];
memcpy(temp, left, BLOCK_SIZE / 2);
memcpy(left, right, BLOCK_SIZE / 2);
memcpy(right, temp, BLOCK_SIZE / 2);
}
}
merge(block, right, left);
}
int main() {
unsigned char block[BLOCK_SIZE] = {0x01, 0x23, 0x45, 0x67, 0x89, 0xAB, 0xCD, 0xEF};
unsigned char key[KEY_SIZE] = {0x12, 0x34, 0x56, 0x78, 0x9A, 0xBC, 0xDE, 0xF0};
feistel_encrypt(block, key);
printf("Encrypted block: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%02X", block[i]);
}
printf("\n");
return 0;
}
```
上述示例代码实现了一个简单的Feistel结构加密算法,其中使用了S盒、P盒和异或操作等基本操作。需要注意的是,该实现仅供学习参考使用,实际应用中需要考虑更多的安全性问题。
相关推荐
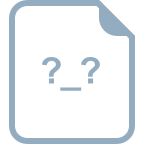
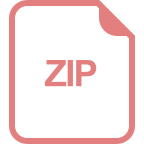
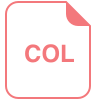














