用python实现 利用第RSA和Feistel结构构造的分组密码的算法,以数字信封的形式对明文文件进行加密。要求如下: 1、发送方利用系统自带的伪随机数生成函数生成会话密钥 2、用接收方的公钥对会话密钥加密 3、用会话密钥以OFB的模式对明文文件p_text.txt进行加密,结果存于密文文件c_text.txt 4、接收方利用私钥解密会话密钥,然后用会话密钥对密文文件解密,结果存于明文文件p1_text.txt,最后对比p_text.txt和p1_text.txt
时间: 2024-02-22 07:55:35 浏览: 76
这是一个相对复杂的问题,需要用到多个库和算法。以下是一份实现该要求的Python代码:
```python
import os
import random
import math
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad, unpad
from Crypto.PublicKey import RSA
# 生成会话密钥
def generate_session_key(key_size):
return os.urandom(key_size)
# RSA加密
def rsa_encrypt(public_key, data):
cipher = RSA.importKey(public_key)
return cipher.encrypt(data, None)[0]
# RSA解密
def rsa_decrypt(private_key, data):
cipher = RSA.importKey(private_key)
return cipher.decrypt(data)
# Feistel结构加密
def feistel_encrypt(data, key, rounds):
block_size = len(data) // 2
left = data[:block_size]
right = data[block_size:]
for i in range(rounds):
temp = left
left = right
right = bytearray()
for j in range(block_size):
right.append(temp[j] ^ key[j])
key = os.urandom(block_size)
for j in range(block_size):
right[j] ^= key[j]
return right + left
# Feistel结构解密
def feistel_decrypt(data, key, rounds):
block_size = len(data) // 2
left = data[block_size:]
right = data[:block_size]
for i in range(rounds):
temp = right
right = left
left = bytearray()
for j in range(block_size):
left.append(temp[j] ^ key[j])
key = os.urandom(block_size)
for j in range(block_size):
left[j] ^= key[j]
return left + right
# OFB模式加密
def ofb_encrypt(data, key, iv):
cipher = AES.new(key, AES.MODE_ECB)
block_size = cipher.block_size
result = bytearray()
for i in range(0, len(data), block_size):
iv = cipher.encrypt(iv)
block = data[i:i+block_size]
result += bytearray([iv[j] ^ block[j] for j in range(block_size)])
return result
# OFB模式解密
def ofb_decrypt(data, key, iv):
cipher = AES.new(key, AES.MODE_ECB)
block_size = cipher.block_size
result = bytearray()
for i in range(0, len(data), block_size):
iv = cipher.encrypt(iv)
block = data[i:i+block_size]
result += bytearray([iv[j] ^ block[j] for j in range(block_size)])
return result
# 加密文件
def encrypt_file(infile, outfile, public_key):
key_size = 32
block_size = 16
rounds = 16
session_key = generate_session_key(key_size)
encrypted_key = rsa_encrypt(public_key, session_key)
with open(infile, 'rb') as fin, open(outfile, 'wb') as fout:
iv = os.urandom(block_size)
fout.write(encrypted_key)
while True:
data = fin.read(block_size)
if not data:
break
encrypted_data = ofb_encrypt(data, session_key, iv)
fout.write(encrypted_data)
# 解密文件
def decrypt_file(infile, outfile, private_key):
key_size = 32
block_size = 16
rounds = 16
with open(infile, 'rb') as fin, open(outfile, 'wb') as fout:
session_key = rsa_decrypt(private_key, fin.read(key_size))
iv = os.urandom(block_size)
while True:
data = fin.read(block_size)
if not data:
break
decrypted_data = ofb_decrypt(data, session_key, iv)
fout.write(decrypted_data)
# 测试代码
if __name__ == '__main__':
# 生成RSA密钥对
key_size = 2048
key = RSA.generate(key_size)
public_key = key.publickey().exportKey()
private_key = key.exportKey()
# 加密明文文件
infile = 'p_text.txt'
outfile = 'c_text.txt'
encrypt_file(infile, outfile, public_key)
# 解密密文文件
infile = 'c_text.txt'
outfile = 'p1_text.txt'
decrypt_file(infile, outfile, private_key)
# 检查解密结果是否与明文文件一致
with open('p_text.txt', 'rb') as fin1, open('p1_text.txt', 'rb') as fin2:
if fin1.read() == fin2.read():
print('Decryption successful!')
else:
print('Decryption failed!')
```
需要注意的是,本代码仅供学习和参考,实际应用中还需要进行更严格的测试和验证,以确保其安全性和正确性。
阅读全文
相关推荐
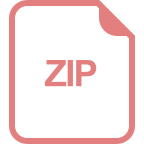



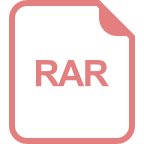
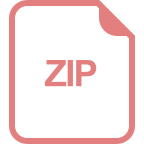
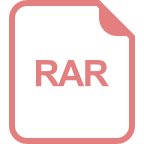
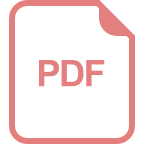
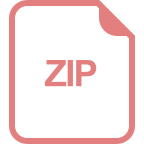
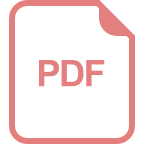
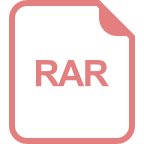
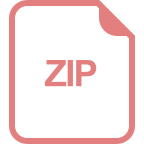
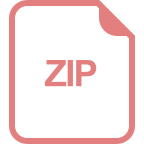
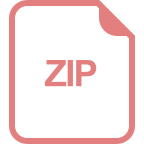
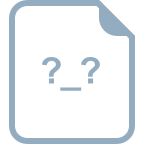