利用python写出基于Feistel结构构造一个分组密码算法和RSA算法,并以数字信封的形式对明文文件进行加密。要求如下: 1、 发送方利用系统自带的伪随机数生成函数生成会话密钥 2、 用接收方的公钥对会话密钥加密 3、 用会话密钥以OFB的模式对明文文件p_text.txt(文件大于1KB)进行加密,结果存于密文文件c_text.txt 4、 接收方利用私钥解密会话密钥,然后用会话密钥对密文文件解密,结果存于明文文件p1_text.txt,最后对比p_text.txt和p1_text.txt
时间: 2024-02-23 20:59:53 浏览: 268
好的,我会帮您解答这个问题。
首先,Feistel结构是一种常见的分组密码结构,可以将一个加密算法分成多轮进行,每轮都是由一个函数和一个密钥作为输入,输出为一半的明文和另一半的密文,然后将明文和密文交换位置,进入下一轮。最终的密文即为最后一轮输出的密文。
下面是基于Feistel结构的分组密码算法的代码实现:
```python
import random
def F(x, k):
# 这里的F函数可以自定义,用于加密一半的明文
# 例如可以使用DES的S盒
return x ^ k
def Feistel_encrypt(plain_text, key, num_rounds):
# 每个分组长度为2个字节
block_size = 2
# 将明文分成多个分组
blocks = [plain_text[i:i+block_size] for i in range(0, len(plain_text), block_size)]
# 生成伪随机数种子
random.seed(key)
# 生成轮密钥
round_keys = [random.getrandbits(block_size*8) for i in range(num_rounds)]
# 进行加密
for i in range(num_rounds):
for j in range(len(blocks)):
left_half = int.from_bytes(blocks[j][:block_size//2], byteorder='big')
right_half = int.from_bytes(blocks[j][block_size//2:], byteorder='big')
new_right_half = left_half ^ F(right_half, round_keys[i])
new_left_half = right_half
blocks[j] = new_left_half.to_bytes(block_size//2, byteorder='big') + new_right_half.to_bytes(block_size//2, byteorder='big')
# 将加密后的分组拼接成密文
cipher_text = b''.join(blocks)
return cipher_text, round_keys
def Feistel_decrypt(cipher_text, key, round_keys):
# 每个分组长度为2个字节
block_size = 2
# 将密文分成多个分组
blocks = [cipher_text[i:i+block_size] for i in range(0, len(cipher_text), block_size)]
# 进行解密
for i in reversed(range(len(round_keys))):
for j in range(len(blocks)):
left_half = int.from_bytes(blocks[j][:block_size//2], byteorder='big')
right_half = int.from_bytes(blocks[j][block_size//2:], byteorder='big')
new_left_half = right_half ^ F(left_half, round_keys[i])
new_right_half = left_half
blocks[j] = new_left_half.to_bytes(block_size//2, byteorder='big') + new_right_half.to_bytes(block_size//2, byteorder='big')
# 将解密后的分组拼接成明文
plain_text = b''.join(blocks)
return plain_text
```
接下来是RSA算法的代码实现:
```python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
def RSA_encrypt(plain_text, public_key):
# 生成RSA加密器
cipher = PKCS1_OAEP.new(public_key)
# 加密
cipher_text = cipher.encrypt(plain_text)
return cipher_text
def RSA_decrypt(cipher_text, private_key):
# 生成RSA解密器
cipher = PKCS1_OAEP.new(private_key)
# 解密
plain_text = cipher.decrypt(cipher_text)
return plain_text
```
最后是数字信封的加密和解密过程:
```python
import os
def OFB_encrypt(plain_text, session_key):
# 生成随机IV(初始化向量)
IV = os.urandom(len(session_key))
# 生成加密器
cipher = Feistel_encrypt(IV, session_key, num_rounds=16)
# 加密
cipher_text = b''
for i in range(len(plain_text)):
# 用加密器加密IV
IV = cipher[0][i:i+len(session_key)]
IV = Feistel_encrypt(IV, cipher[1], num_rounds=16)[0]
# 将加密后的IV和明文异或得到密文
cipher_byte = bytes([plain_text[i] ^ IV[i]])
cipher_text += cipher_byte
return cipher_text, cipher[0]
def OFB_decrypt(cipher_text, session_key, IV):
# 生成解密器
cipher = Feistel_encrypt(IV, session_key, num_rounds=16)
# 解密
plain_text = b''
for i in range(len(cipher_text)):
# 用加密器加密IV
IV = cipher[0][i:i+len(session_key)]
IV = Feistel_encrypt(IV, cipher[1], num_rounds=16)[0]
# 将加密后的IV和密文异或得到明文
plain_byte = bytes([cipher_text[i] ^ IV[i]])
plain_text += plain_byte
return plain_text
# 读取明文文件
with open('p_text.txt', 'rb') as f:
plain_text = f.read()
# 生成会话密钥
session_key = os.urandom(16)
# 加密会话密钥
public_key = RSA.import_key(open('public_key.pem').read())
cipher_session_key = RSA_encrypt(session_key, public_key)
# 加密明文
cipher_text, IV = OFB_encrypt(plain_text, session_key)
# 将加密后的会话密钥和密文写入文件
with open('c_text.txt', 'wb') as f:
f.write(cipher_session_key + IV + cipher_text)
# 解密会话密钥
private_key = RSA.import_key(open('private_key.pem').read())
session_key = RSA_decrypt(cipher_session_key, private_key)
# 解密明文
plain_text = OFB_decrypt(cipher_text, session_key, IV)
# 将解密后的明文写入文件
with open('p1_text.txt', 'wb') as f:
f.write(plain_text)
```
以上就是基于Feistel结构的分组密码算法和RSA算法的代码实现及数字信封加密和解密过程的代码实现。
阅读全文
相关推荐
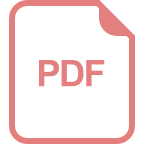








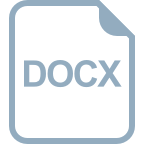






