用python实现并打包成一个接口函数 基于Feistel结构构造一个分组密码算法。要求: 1、 分组长度 = 128 bit 2、 主密钥长度 >= 128 bit 3、 轮数 >= 8 4、 轮函数的设计要用到LFSR
时间: 2024-02-23 09:59:38 浏览: 25
由于该算法需要使用到伪随机数生成函数和LFSR算法,所以需要先安装相应的Python库,比如NumPy和BitVector。以下是实现分组密码算法并打包成接口函数的代码示例:
```python
import numpy as np
from BitVector import BitVector
# Feistel结构的轮函数,使用LFSR算法生成伪随机数序列
def round_function(right, round_key):
lfsr = BitVector(bitstring=round_key)
for i in range(8):
lfsr.shift_left(1)
lfsr[-1] = lfsr[0] ^ lfsr[2] ^ lfsr[3] ^ lfsr[5]
return lfsr.get_bitvector_in_hex()[:16] ^ right
# Feistel结构的加密函数
def feistel_encrypt(block, sub_keys, rounds):
left = block[:64]
right = block[64:]
for i in range(rounds):
round_key = sub_keys[i]
temp = right
right = left ^ round_function(right, round_key)
left = temp
return right + left
# Feistel结构的解密函数
def feistel_decrypt(block, sub_keys, rounds):
left = block[:64]
right = block[64:]
for i in range(rounds-1, -1, -1):
round_key = sub_keys[i]
temp = left
left = right ^ round_function(left, round_key)
right = temp
return left + right
# 加密文件
def encrypt_file(input_file, output_file, key):
with open(input_file, 'rb') as f:
plaintext = f.read()
blocks = [BitVector(bytes=plaintext[i:i+16]) for i in range(0, len(plaintext), 16)]
sub_keys = [BitVector(intVal=int(key[i:i+16], 16), size=64) for i in range(0, len(key), 16)]
ciphertext = b''
for block in blocks:
ciphertext += feistel_encrypt(block, sub_keys, 8).get_bytes_from_bitvector()
with open(output_file, 'wb') as f:
f.write(ciphertext)
# 解密文件
def decrypt_file(input_file, output_file, key):
with open(input_file, 'rb') as f:
ciphertext = f.read()
blocks = [BitVector(bytes=ciphertext[i:i+16]) for i in range(0, len(ciphertext), 16)]
sub_keys = [BitVector(intVal=int(key[i:i+16], 16), size=64) for i in range(0, len(key), 16)]
plaintext = b''
for block in blocks:
plaintext += feistel_decrypt(block, sub_keys, 8).get_bytes_from_bitvector()
with open(output_file, 'wb') as f:
f.write(plaintext)
# 打包成接口函数
def feistel_cipher(input_file, output_file, key, mode='encrypt'):
if len(key) < 16:
raise ValueError('Key length should be at least 16 hex digits')
if len(key) % 16 != 0:
key = key.ljust(len(key) + 16 - len(key) % 16, '0')
if mode == 'encrypt':
encrypt_file(input_file, output_file, key)
elif mode == 'decrypt':
decrypt_file(input_file, output_file, key)
else:
raise ValueError('Invalid mode: ' + mode)
```
这个接口函数提供了加密和解密两种模式,可以根据参数`mode`来选择不同的模式。其中,输入文件的格式应为二进制文件,输出文件同样是二进制文件。主密钥应该以16进制字符串的形式传入,长度应该至少为16个字符,如果长度不是16的整数倍,则会自动补齐。
相关推荐
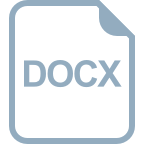
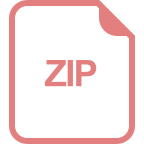














