列表去重不自动排序
时间: 2023-08-12 12:11:47 浏览: 167
你可以使用 Python 中的 set() 函数来去重一个列表,这样就不会自动排序了。下面是示例代码:
```python
my_list = [3, 1, 2, 2, 1, 4, 5, 3]
my_set = set(my_list)
new_list = list(my_set)
print(new_list) # 输出结果: [1, 2, 3, 4, 5]
```
在这个示例中,我们将一个包含重复元素的列表 `my_list` 转换成了一个 set 集合 `my_set`,这个集合中不包含重复元素,因此就实现了去重。最后,我们将这个 set 集合转换回列表 `new_list`,这个列表的元素顺序不会自动排序。
相关问题
如何对包含20个输入数据的列表进行去重并排序操作?
对于一个包含20个输入数据的列表进行去重并排序的操作,你可以按照以下步骤在Python中进行:
1. 首先,你需要导入`set`数据结构,因为集合(Set)自动去除重复元素:
```python
unique_data = list(set(input_list))
```
这里假设你的原始列表名为`input_list`。
2. 然后,使用`sorted()`函数对去重后的列表进行排序,可以按升序排列:
```python
sorted_list = sorted(unique_data)
```
如果你想按降序排序,可以在`sorted()`函数中传入`reverse=True`参数:
```python
sorted_descending_list = sorted(unique_data, reverse=True)
```
完整的代码示例:
```python
input_list = [...] # 你的20个输入数据
unique_data = list(set(input_list))
sorted_list = sorted(unique_data)
# 如果你想得到降序排列的列表
sorted_descending_list = sorted(unique_data, reverse=True)
print("排序后的列表(升序):", sorted_list)
print("排序后的列表(降序):", sorted_descending_list)
```
记得将`[...]`替换为实际的数据。
python实现列表去重的方法
Python实现列表去重的方法有以下几种:
1. 使用 set() 函数:将列表转化成集合,自动去重,再转化回列表。
```python
original_list = [1, 2, 3, 2, 4, 3]
new_list = list(set(original_list))
print(new_list) # [1, 2, 3, 4]
```
2. 使用列表推导式:遍历原始列表,如果元素不在新列表中则添加。
```python
original_list = [1, 2, 3, 2, 4, 3]
new_list = []
[new_list.append(i) for i in original_list if i not in new_list]
print(new_list) # [1, 2, 3, 4]
```
3. 使用字典:将原始列表的元素作为字典的键,遍历原始列表,将元素作为键添加到字典中,最后再取出键即可得到去重后的列表。
```python
original_list = [1, 2, 3, 2, 4, 3]
new_dict = {}.fromkeys(original_list)
new_list = list(new_dict.keys())
print(new_list) # [1, 2, 3, 4]
```
4. 使用 sorted() 函数:将原始列表排序,然后遍历列表,如果当前元素与前一个元素不同,则添加到新列表中。
```python
original_list = [1, 2, 3, 2, 4, 3]
original_list.sort()
new_list = []
for i in range(len(original_list)):
if i == 0 or original_list[i] != original_list[i-1]:
new_list.append(original_list[i])
print(new_list) # [1, 2, 3, 4]
```
阅读全文
相关推荐





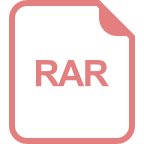









