C++,list去重
时间: 2023-11-15 08:59:00 浏览: 152
C++中可以使用STL中的set或者unordered_set来实现list去重。其中set是基于红黑树实现的,可以自动排序,而unordered_set是基于哈希表实现的,查找速度更快。具体实现方法如下:
1.使用set实现list去重:
```
#include <iostream>
#include <list>
#include <set>
using namespace std;
int main()
{
list<int> myList = {1, 2, 3, 2, 4, 5, 3};
set<int> mySet(myList.begin(), myList.end());
myList.assign(mySet.begin(), mySet.end());
for (auto i : myList)
{
cout << i << " ";
}
return 0;
}
```
2.使用unordered_set实现list去重:
```
#include <iostream>
#include <list>
#include <unordered_set>
using namespace std;
int main()
{
list<int> myList = {1, 2, 3, 2, 4, 5, 3};
unordered_set<int> mySet(myList.begin(), myList.end());
myList.assign(mySet.begin(), mySet.end());
for (auto i : myList)
{
cout << i << " ";
}
return 0;
}
```
阅读全文
相关推荐






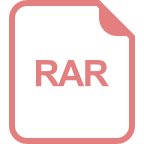











