ValueError: SHA could not be resolved, git returned: b''
时间: 2024-05-16 16:17:33 浏览: 214
这个错误通常表示 Git 无法解析 SHA 标识符。这可能是由于您在 Git 存储库中提供了无效的 SHA 标识符,或者是由于您的 Git 存储库已损坏。
您可以尝试以下步骤来解决该问题:
1. 确保您提供的 SHA 标识符是有效的。您可以通过运行 `git show <SHA>` 来检查 SHA 标识符是否有效。
2. 如果您确定 SHA 标识符是有效的,请尝试从本地备份中恢复 Git 存储库。您可以使用以下命令从本地备份中恢复 Git 存储库:
```
git clone --mirror /path/to/local/backup.git /path/to/new/repository.git
```
这将从本地备份中创建一个新的 Git 存储库。
3. 如果以上步骤都无法解决问题,请尝试重新克隆 Git 存储库。您可以使用以下命令重新克隆 Git 存储库:
```
git clone <repository-url>
```
这将从头开始克隆 Git 存储库。请注意,这将删除当前存储库中所有未提交的更改。
相关问题
ValueError: operands could not be broadcast together with shape (2400,) (2,)
This error occurs when trying to perform an operation on two arrays with incompatible shapes. In this case, the first array has a shape of (2400,) and the second array has a shape of (2,).
To fix this error, you need to make sure that the shapes of the two arrays are compatible for the operation you are trying to perform. You can reshape one or both of the arrays to match the desired shape, or you can use broadcasting to perform the operation.
For example, if you want to add the two arrays element-wise, you can reshape the second array to have the same shape as the first array:
```
import numpy as np
a = np.random.rand(2400)
b = np.array([1, 2])
b = np.tile(b, 1200) # repeat b 1200 times to match the shape of a
c = a + b
```
Alternatively, you can use broadcasting to add the second array to each element of the first array:
```
import numpy as np
a = np.random.rand(2400)
b = np.array([1, 2])
c = a + b[:, np.newaxis] # add b to each element of a
```
In this case, broadcasting automatically expands the second array to have the same shape as the first array, allowing the addition to be performed element-wise.
ValueError: operands could not be broadcast together with shapes (2,) (100,)
在Python中,当你尝试对两个数组进行操作时,如果它们的形状不兼容,就会出现"ValueError: operands could not be broadcast together with shapes"错误。这个错误通常发生在你尝试对形状不同的数组进行元素级别的操作时,比如加法、减法、乘法等。
要解决这个错误,你可以考虑以下几种方法:
1. 确保数组的形状相同:你可以使用NumPy库的reshape()函数来调整数组的形状,使其与另一个数组的形状相匹配。例如,如果你有一个形状为(2,)的数组和一个形状为(100,)的数组,你可以使用reshape()函数将第一个数组的形状调整为(2,1),然后再进行操作。
```python
import numpy as np
array1 = np.array([1, 2])
array2 = np.array([1, 2, 3, ..., 100])
# 调整数组形状
array1_reshaped = array1.reshape((2, 1))
# 进行操作
result = array1_reshaped + array2
```
2. 使用广播功能:NumPy库提供了广播功能,可以自动调整数组的形状,使其与另一个数组的形状相匹配。你只需要确保数组的维度兼容即可。例如,如果你有一个形状为(2,)的数组和一个形状为(100,)的数组,你可以使用广播功能直接进行操作。
```python
import numpy as np
array1 = np.array([1, 2])
array2 = np.array([1, 2, 3, ..., 100])
# 进行操作
result = array1 + array2
```
3. 检查数组的形状:在出现这个错误时,你可以检查一下两个数组的形状,确保它们是你期望的形状。你可以使用NumPy库的shape属性来获取数组的形状。
```python
import numpy as np
array1 = np.array([1, 2])
array2 = np.array([1, 2, 3, ..., 100])
# 检查数组形状
print(array1.shape) # 输出:(2,)
print(array2.shape) # 输出:(100,)
```
阅读全文
相关推荐
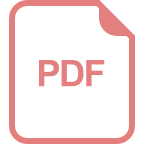
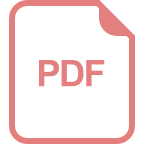
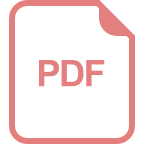













