请用Servlet加JavaBean加jsp文件完整实现复数加减乘除四则运算
时间: 2024-02-23 20:01:49 浏览: 25
好的,以下是完整实现复数加减乘除四则运算的代码:
1. JavaBean
```
public class Complex {
private double real;
private double imag;
public Complex() {
this.real = 0;
this.imag = 0;
}
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public double getReal() {
return real;
}
public void setReal(double real) {
this.real = real;
}
public double getImag() {
return imag;
}
public void setImag(double imag) {
this.imag = imag;
}
public Complex add(Complex b) {
Complex a = this;
double real = a.real + b.real;
double imag = a.imag + b.imag;
return new Complex(real, imag);
}
public Complex subtract(Complex b) {
Complex a = this;
double real = a.real - b.real;
double imag = a.imag - b.imag;
return new Complex(real, imag);
}
public Complex multiply(Complex b) {
Complex a = this;
double real = a.real * b.real - a.imag * b.imag;
double imag = a.real * b.imag + a.imag * b.real;
return new Complex(real, imag);
}
public Complex divide(Complex b) {
Complex a = this;
double real = (a.real * b.real + a.imag * b.imag) / (b.real * b.real + b.imag * b.imag);
double imag = (a.imag * b.real - a.real * b.imag) / (b.real * b.real + b.imag * b.imag);
return new Complex(real, imag);
}
}
```
2. Servlet
```
@WebServlet("/ComplexCalculator")
public class ComplexCalculator extends HttpServlet {
private static final long serialVersionUID = 1L;
public ComplexCalculator() {
super();
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
double real1 = Double.parseDouble(request.getParameter("real1"));
double imag1 = Double.parseDouble(request.getParameter("imag1"));
double real2 = Double.parseDouble(request.getParameter("real2"));
double imag2 = Double.parseDouble(request.getParameter("imag2"));
Complex c1 = new Complex(real1, imag1);
Complex c2 = new Complex(real2, imag2);
Complex result = null;
String operator = request.getParameter("operator");
switch (operator) {
case "+":
result = c1.add(c2);
break;
case "-":
result = c1.subtract(c2);
break;
case "*":
result = c1.multiply(c2);
break;
case "/":
result = c1.divide(c2);
break;
default:
break;
}
request.setAttribute("result", result);
RequestDispatcher dispatcher = request.getRequestDispatcher("result.jsp");
dispatcher.forward(request, response);
}
}
```
3. JSP
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Complex Calculator</title>
</head>
<body>
<h1>Complex Calculator</h1>
<form method="post" action="ComplexCalculator">
<input type="number" name="real1" step="0.01" required> + <input type="number" name="imag1" step="0.01" required> i
<select name="operator">
<option value="+">+</option>
<option value="-">-</option>
<option value="*">*</option>
<option value="/">/</option>
</select>
<input type="number" name="real2" step="0.01" required> + <input type="number" name="imag2" step="0.01" required> i
<input type="submit" value="Calculate">
</form>
<%
Complex result = (Complex) request.getAttribute("result");
if (result != null) {
out.println("<p>Result: " + result.getReal() + " + " + result.getImag() + "i</p>");
}
%>
</body>
</html>
```
以上是完整的实现代码,可以实现复数的加减乘除四则运算。
相关推荐
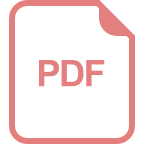














