现有A、B两个线程,文件夹S中有10张图片,图片名分别为a1.jpg或a1.png、b2.jpg或b2.png、c3..d4..以此类推..k10.jpg或k10.png,A线程任务是往文件夹D中拷贝图片,B线程任务是每有一张图片拷贝进来,需将图片名称改成java
时间: 2024-02-06 07:11:01 浏览: 76
可以使用Java中的多线程和文件操作相关的API来实现该功能。以下是一个可能的实现:
```java
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ImageCopyAndRename {
// 源图片文件夹路径
private static final String SOURCE_PATH = "S";
// 目标图片文件夹路径
private static final String DEST_PATH = "D";
// 时间戳格式
private static final SimpleDateFormat TIMESTAMP_FORMAT = new SimpleDateFormat("yyyyMMddHHmmssSSS");
public static void main(String[] args) {
// 创建线程池
ExecutorService executor = Executors.newFixedThreadPool(2);
// 遍历源图片文件夹
File sourceDir = new File(SOURCE_PATH);
if (sourceDir.exists() && sourceDir.isDirectory()) {
File[] files = sourceDir.listFiles();
if (files != null && files.length > 0) {
for (File file : files) {
// A线程:拷贝图片到目标文件夹
executor.execute(() -> copyImage(file));
// B线程:每有一张图片拷贝进来,改名后放到目标文件夹
executor.execute(() -> renameImage(file));
}
}
}
// 关闭线程池
executor.shutdown();
}
// 拷贝图片到目标文件夹
private static void copyImage(File sourceFile) {
Path sourcePath = Paths.get(sourceFile.getAbsolutePath());
Path destPath = Paths.get(DEST_PATH, sourceFile.getName());
try {
Files.copy(sourcePath, destPath);
System.out.println("Copied " + sourceFile.getName() + " to " + destPath.toString());
} catch (IOException e) {
System.err.println("Failed to copy " + sourceFile.getName() + ": " + e.getMessage());
}
}
// 改名后放到目标文件夹
private static void renameImage(File sourceFile) {
Path sourcePath = Paths.get(sourceFile.getAbsolutePath());
String timestamp = TIMESTAMP_FORMAT.format(new Date());
String newName = timestamp + sourceFile.getName();
Path destPath = Paths.get(DEST_PATH, newName);
try {
Files.move(sourcePath, destPath);
System.out.println("Renamed and moved " + sourceFile.getName() + " to " + destPath.toString());
} catch (IOException e) {
System.err.println("Failed to rename and move " + sourceFile.getName() + ": " + e.getMessage());
}
}
}
```
该程序首先创建一个线程池,然后遍历源图片文件夹,对于每个图片文件,分别启动A线程和B线程。
A线程使用`Files.copy`方法将图片文件拷贝到目标文件夹。
B线程先生成一个时间戳,然后将时间戳和原文件名拼接成新的文件名,使用`Files.move`方法将图片文件重命名并移动到目标文件夹。
值得注意的是,该程序中的代码没有处理文件名重复的情况。如果源文件夹中存在重名的文件,或者同时有多个文件拷贝到目标文件夹并且生成了相同的时间戳,就会导致重名文件的覆盖。如果需要处理这种情况,可以在B线程中使用循环来不断尝试生成不重复的文件名。
阅读全文
相关推荐





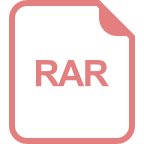







