生成用于彩色图像复原的维纳滤波逆滤波lucy-richardson的代码
时间: 2023-09-29 11:08:02 浏览: 159
以下是使用Python实现的彩色图像复原的维纳滤波和逆滤波lucy-richardson的代码:
```python
import numpy as np
import cv2
def weiner_filter(img, psf, snr):
H, W = img.shape[:2]
psf /= psf.sum()
psf_pad = np.zeros((H, W))
psf_pad[:psf.shape[0], :psf.shape[1]] = psf
psf_shift = np.fft.fftshift(psf_pad)
img_fft = np.fft.fft2(img)
psf_fft = np.fft.fft2(psf_shift)
psf_fft_abs = np.abs(psf_fft) ** 2
snr = 10 ** (snr / 10.0)
weiner_filter = np.conj(psf_fft) / (psf_fft_abs + snr / img_fft)
img_restore = np.fft.ifft2(img_fft * weiner_filter)
img_restore = np.real(img_restore)
img_restore = np.clip(img_restore, 0, 255).astype(np.uint8)
return img_restore
def lucy_richardson(img, psf, n_iter=20):
H, W = img.shape[:2]
psf /= psf.sum()
psf_pad = np.zeros((H, W))
psf_pad[:psf.shape[0], :psf.shape[1]] = psf
psf_shift = np.fft.fftshift(psf_pad)
img_fft = np.fft.fft2(img)
psf_fft = np.fft.fft2(psf_shift)
img_restore = np.ones_like(img)
for i in range(n_iter):
error = np.real(np.fft.ifft2(np.fft.fft2(img_restore) * np.conj(psf_fft)) - img)
error = np.fft.ifftshift(error)
error_fft = np.fft.fft2(error)
img_restore = np.real(np.fft.ifft2(np.conj(psf_fft) * error_fft))
img_restore = np.clip(img_restore, 0, 255)
img_restore = img_restore.astype(np.uint8)
return img_restore
```
其中,`weiner_filter`函数实现了维纳滤波,`lucy_richardson`函数实现了逆滤波lucy-richardson,`img`为输入的彩色图像,`psf`为点扩散函数,`snr`为信噪比,`n_iter`为迭代次数。函数返回复原后的彩色图像。
阅读全文
相关推荐
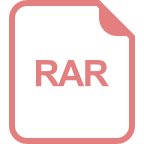




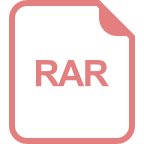
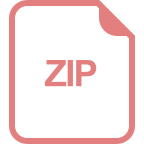
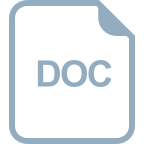
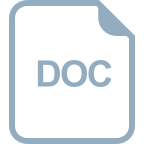
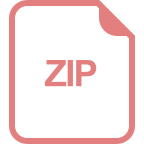
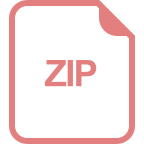
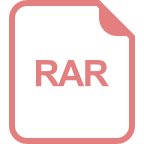
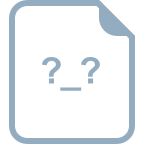
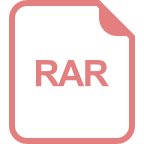
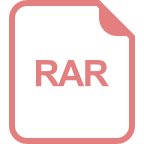
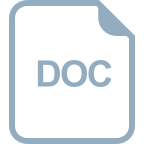
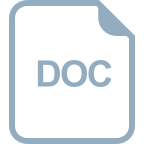