请为我写一段精准度比较高的LSTM回归预测模型,并对结果可视化。
时间: 2024-03-26 10:41:32 浏览: 14
好的,下面是一个简单的LSTM回归预测模型的示例代码,包括数据处理、模型构建、训练和可视化等步骤。
首先,我们生成一些假数据,用于模型训练和测试:
```python
import numpy as np
# 生成随机数据
np.random.seed(0)
X = np.random.rand(1000, 1)
y = np.sin(X * 10) + np.random.randn(1000, 1) * 0.1
```
接下来,我们将数据划分为训练集和测试集,并进行归一化处理:
```python
from sklearn.preprocessing import StandardScaler, MinMaxScaler, MaxAbsScaler
from sklearn.model_selection import train_test_split
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
# 归一化处理
scaler_x = MinMaxScaler()
X_train = scaler_x.fit_transform(X_train)
X_test = scaler_x.transform(X_test)
scaler_y = MinMaxScaler()
y_train = scaler_y.fit_transform(y_train)
y_test = scaler_y.transform(y_test)
```
接下来,我们构建LSTM回归预测模型。在这个模型中,我们使用了一个LSTM层和一个全连接层,其中LSTM层的输出被传递给全连接层,最终输出一个标量值。
```python
import torch
import torch.nn as nn
class LSTMRegressor(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super().__init__()
self.lstm = nn.LSTM(input_size, hidden_size, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
lstm_out, _ = self.lstm(x)
y_pred = self.fc(lstm_out[:, -1, :])
return y_pred
```
接下来,我们定义一些模型超参数:
```python
# 定义超参数
input_size = 1
hidden_size = 32
output_size = 1
learning_rate = 0.001
num_epochs = 1000
```
然后,我们创建模型、定义损失函数和优化器:
```python
# 创建模型
model = LSTMRegressor(input_size, hidden_size, output_size)
# 定义损失函数和优化器
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=learning_rate)
```
接下来,我们进行模型训练:
```python
# 开始训练模型
for epoch in range(num_epochs):
# 将数据转换为Tensor
inputs = torch.Tensor(X_train).unsqueeze(2)
labels = torch.Tensor(y_train).unsqueeze(1)
# 前向传播
outputs = model(inputs)
loss = criterion(outputs, labels)
# 反向传播
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 输出训练结果
if (epoch+1) % 100 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
```
最后,我们对模型进行测试,并可视化预测结果:
```python
import matplotlib.pyplot as plt
# 对模型进行测试
model.eval()
with torch.no_grad():
inputs = torch.Tensor(X_test).unsqueeze(2)
labels = torch.Tensor(y_test).unsqueeze(1)
outputs = model(inputs)
loss = criterion(outputs, labels)
print('Test Loss: {:.4f}'.format(loss.item()))
# 可视化结果
plt.figure(figsize=(10, 5))
plt.plot(X_test, y_test, label='Ground Truth')
plt.plot(X_test, outputs.numpy(), label='Predictions')
plt.legend(loc='upper left')
plt.show()
```
这里我们使用了Matplotlib库进行可视化,可以将模型预测结果与真实数据进行比较。
希望这个示例代码可以对你有所帮助!
相关推荐
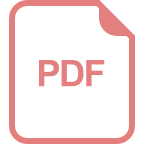
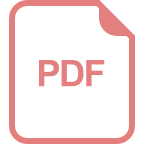
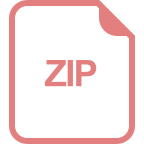













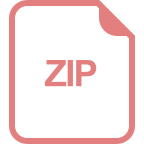
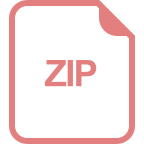