写一个untiy批量识别球体位置并以此位置来批量渲染全景图的插件
时间: 2024-02-11 16:06:07 浏览: 94
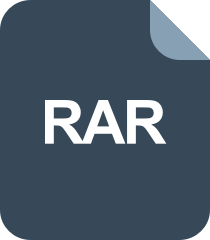
全景图插件
好的,我可以为您提供一些思路和大致的实现方式。
首先,您需要编写一个脚本来识别球体的位置。您可以使用Unity的物理引擎来检测球体是否与其他物体碰撞,通过这种方式来获取球体的位置。
接下来,您需要编写一个批量渲染全景图的脚本。您可以使用Unity的RenderTexture功能来创建全景图,并将相机的位置和旋转信息应用到RenderTexture上,从而实现批量渲染全景图。
最后,将这两个脚本结合起来。在检测到球体位置后,将相机移动到球体的位置,并使用批量渲染全景图的脚本来渲染全景图。
以下是一个简单的示例代码:
```csharp
using UnityEngine;
public class BatchRenderPlugin : MonoBehaviour
{
public GameObject[] spheres; // 所有球体对象
public Camera camera; // 渲染用相机
private RenderTexture renderTexture; // 渲染纹理
void Start()
{
renderTexture = new RenderTexture(Screen.width, Screen.height, 24);
camera.targetTexture = renderTexture;
}
void Update()
{
// 循环所有球体
foreach (GameObject sphere in spheres)
{
// 获取球体位置
Vector3 spherePos = sphere.transform.position;
// 将相机移动到球体位置
camera.transform.position = spherePos;
// 渲染全景图
camera.Render();
// 保存全景图
string fileName = "sphere_" + sphere.name + ".png";
SaveRenderTextureToFile(renderTexture, fileName);
}
}
// 将渲染纹理保存到文件
void SaveRenderTextureToFile(RenderTexture rt, string fileName)
{
Texture2D tex = new Texture2D(rt.width, rt.height, TextureFormat.RGB24, false);
RenderTexture.active = rt;
tex.ReadPixels(new Rect(0, 0, rt.width, rt.height), 0, 0);
tex.Apply();
byte[] bytes = tex.EncodeToPNG();
System.IO.File.WriteAllBytes(Application.dataPath + "/" + fileName, bytes);
}
}
```
以上代码仅供参考,具体实现方式可能需要根据您的需求进行调整。
阅读全文
相关推荐
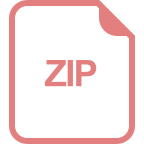
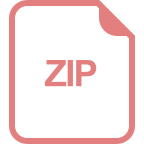
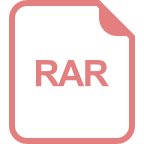
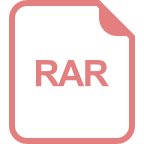









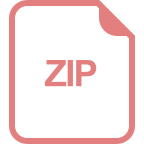