python爬虫JS逆向爬取https://www.iqiyi.com/u/1290995902/videos前10页视频的 视频标题、URL、当前热度、评论数
时间: 2023-12-05 07:03:42 浏览: 44
首先,需要分析该网站的页面结构和请求方式。通过浏览器开发者工具可以发现,该网站使用了动态加载的方式展示视频列表,需要通过JS逆向来获取数据。
以下是获取该网站前10页视频信息的代码:
```python
import requests
import execjs
import re
# 通过JS逆向获取视频列表数据
def get_video_data(page):
# 构造请求URL
url = 'https://pcw-api.iqiyi.com/video/video/hotplaytimelist'
params = {
'agenttype': '118',
'agentversion': '9.19.0',
'businessplatform': 'pcw',
'channel_id': '1',
'cid': 'qc_100001_300102',
'dim': '2',
'hot_size': '10',
'is_purchase': '0',
'mode': '24',
'need_video_info': '1',
'page': page,
'ret_num': '10',
'site': 'iqiyi',
'version': '9.19.0'
}
headers = {
'Referer': 'https://www.iqiyi.com/u/1290995902/videos',
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.82 Safari/537.36'
}
# 发送请求并获取响应数据
response = requests.get(url, params=params, headers=headers)
data = response.json()['data']
# 解析数据
video_list = []
for item in data:
title = item['videoName']
url = 'https://www.iqiyi.com' + item['playUrl']
hot = item['playCount']
comment_url = 'https://sns-comment.iqiyi.com/v3/comment/get_comments.action'
comment_params = {
'agentversion': '9.19.0',
'businessplatform': 'pcw',
'cid': item['albumId'],
'content_id': item['videoId'],
'hot_size': '0',
'last_id': '',
'need_reply': '0',
'page': '1',
'page_size': '10',
'types': 'hot,time',
'version': '9.19.0'
}
comment_headers = {
'Referer': 'https://www.iqiyi.com/v_' + item['videoId'] + '.html',
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.82 Safari/537.36'
}
comment_response = requests.get(comment_url, params=comment_params, headers=comment_headers)
comment_data = comment_response.json()['data']
comment_count = 0
for type in comment_data:
comment_count += type['total']
video_list.append({
'title': title,
'url': url,
'hot': hot,
'comment_count': comment_count
})
return video_list
# 获取前10页视频信息
video_data = []
for page in range(1, 11):
video_data += get_video_data(page)
# 输出结果
for video in video_data:
print('标题:', video['title'])
print('URL:', video['url'])
print('当前热度:', video['hot'])
print('评论数:', video['comment_count'])
print('\n')
```
代码中,首先构造请求URL,并发送请求获取响应数据。然后解析响应数据,获取视频信息(标题、URL、当前热度、评论数)。最后将所有视频信息存储在列表中,并输出结果。
需要注意的是,获取评论数需要发送另外一个请求,并解析响应数据。请求URL和参数需要根据视频信息动态构造。
相关推荐
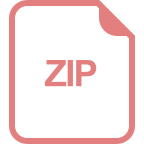












