vue+django聊天室信息的加解密代码
时间: 2023-12-16 18:52:39 浏览: 166
以下是基于Vue.js和Django的聊天室信息的加解密代码示例:
加密代码(Vue.js):
```javascript
import CryptoJS from 'crypto-js';
const secretKey = 'your_secret_key';
function encryptMessage(message) {
const encrypted = CryptoJS.AES.encrypt(message, secretKey);
return encrypted.toString();
}
export default {
encryptMessage,
};
```
解密代码(Django):
```python
import base64
import json
from Crypto.Cipher import AES
class AESCipher:
def __init__(self, key):
self.key = key.encode('utf-8')
def encrypt(self, message):
message = message.encode('utf-8')
cipher = AES.new(self.key, AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(message)
nonce = cipher.nonce
return base64.b64encode(json.dumps({
'nonce': base64.b64encode(nonce).decode('utf-8'),
'ciphertext': base64.b64encode(ciphertext).decode('utf-8'),
'tag': base64.b64encode(tag).decode('utf-8'),
}).encode('utf-8')).decode('utf-8')
def decrypt(self, ciphertext):
data = json.loads(base64.b64decode(ciphertext.encode('utf-8')).decode('utf-8'))
nonce = base64.b64decode(data['nonce'].encode('utf-8'))
ciphertext = base64.b64decode(data['ciphertext'].encode('utf-8'))
tag = base64.b64decode(data['tag'].encode('utf-8'))
cipher = AES.new(self.key, AES.MODE_EAX, nonce=nonce)
plaintext = cipher.decrypt_and_verify(ciphertext, tag)
return plaintext.decode('utf-8')
```
使用示例:
```javascript
import encryptor from '@/utils/encryptor.js';
// 加密消息
const encryptedMessage = encryptor.encryptMessage(message);
// 发送加密消息到 Django 后端
axios.post('/api/messages/', {
message: encryptedMessage,
});
```
```python
from utils.aes_cipher import AESCipher
# 解密消息
cipher = AESCipher('your_secret_key')
decrypted_message = cipher.decrypt(request.data['message'])
# 保存解密后的消息到数据库
Message.objects.create(
content=decrypted_message,
sender=request.user,
)
```
阅读全文
相关推荐
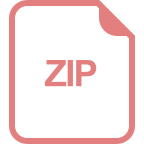
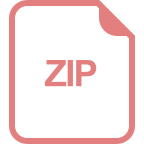
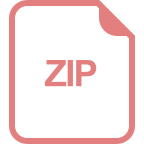
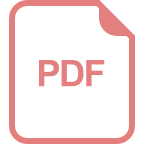
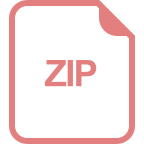
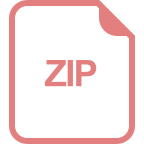
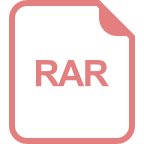
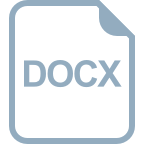
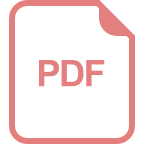
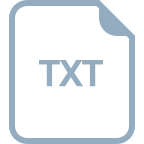
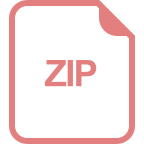
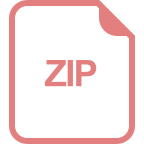
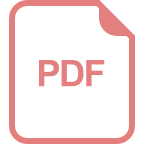
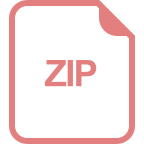
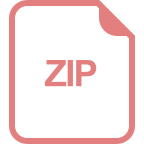
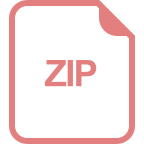
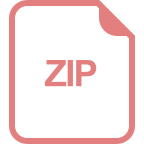