educoder字符串处理
时间: 2023-10-29 13:07:11 浏览: 41
字符串处理在编程中是非常常见的操作,包括字符串拼接、切割、替换、查找等等。在 Educoder 上,可以使用 Python、Java、C++等编程语言进行字符串处理。以下是一些常见的字符串处理操作的实现示例:
Python:
1. 字符串拼接:
```python
str1 = "hello"
str2 = "world"
str3 = str1 + str2
print(str3)
```
2. 字符串切割:
```python
str = "hello,world"
arr = str.split(",")
print(arr)
```
3. 字符串替换:
```python
str = "hello,world"
new_str = str.replace(",", " ")
print(new_str)
```
4. 字符串查找:
```python
str = "hello,world"
index = str.find("world")
print(index)
```
Java:
1. 字符串拼接:
```java
String str1 = "hello";
String str2 = "world";
String str3 = str1 + str2;
System.out.println(str3);
```
2. 字符串切割:
```java
String str = "hello,world";
String[] arr = str.split(",");
System.out.println(Arrays.toString(arr));
```
3. 字符串替换:
```java
String str = "hello,world";
String newStr = str.replace(",", " ");
System.out.println(newStr);
```
4. 字符串查找:
```java
String str = "hello,world";
int index = str.indexOf("world");
System.out.println(index);
```
C++:
1. 字符串拼接:
```cpp
string str1 = "hello";
string str2 = "world";
string str3 = str1 + str2;
cout << str3 << endl;
```
2. 字符串切割:
```cpp
string str = "hello,world";
vector<string> arr;
stringstream ss(str);
string token;
while (getline(ss, token, ',')) {
arr.push_back(token);
}
for (string s : arr) {
cout << s << " ";
}
cout << endl;
```
3. 字符串替换:
```cpp
string str = "hello,world";
replace(str.begin(), str.end(), ',', ' ');
cout << str << endl;
```
4. 字符串查找:
```cpp
string str = "hello,world";
size_t index = str.find("world");
cout << index << endl;
```
相关推荐
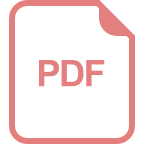














