#include <glut.h> #include <stdio.h> #include <stdlib.h> #define LEFT_EDGE 1 #define RIGHT_EDGE 2 #define BOTTOM_EDGE 4 #define TOP_EDGE 8 struct Rectangle { float xmin, xmax, ymin, ymax; }; Rectangle rect; int x0, y0, x1, y1; void LineGL(int x0, int y0, int x1, int y1) { glBegin(GL_LINES); glColor3f(1.0f, 0.0f, 0.0f); glVertex2f(x0, y0); glColor3f(0.0f, 1.0f, 0.0f); glVertex2f(x1, y1); glEnd(); } //求出坐标点的Cohen-SutherLand编码 int CompCode(int x, int y, Rectangle rect) { int code = 0000; if (y < rect.ymin) code = code | 4; else if (y > rect.ymax) code = code | 8; else if (x < rect.xmin) code = code | 1; else if (x < rect.xmax) code = code | 2; return code; } int cohenSutherland(Rectangle rect, int &x0, int & y0, int &x1, int &y1) { if (CompCode(x,y,rect) & LEFT_EDGE) { y = y0 + (y1 - y0) * (rect.xmin - x0) / (x1 - x0); x = (float)rect.xmin; } return 0; } void Display() { glClear(GL_COLOR_BUFFER_BIT); glColor3f(0.5f, 0.0f, 0.0f); glRectf(rect.xmin, rect.ymin, rect.xmax, rect.ymax); LineGL(x0, y0, x1, y1); glFlush(); } void Init() { glClearColor(0.0, 0.0, 0.0, 0.0); glShadeModel(GL_FLAT); //设定要裁剪的直线和用于裁剪的矩形 rect.xmin = 100; rect.xmax = 500; rect.ymin = 100; rect.ymax = 400; x0 = 0, y0 = 0, x1 = 600, y1 = 300; printf("Press key 'c' to Clip!\nPress key 'r' to Restore!\n"); } void Reshape(int w, int h) { glViewport(0, 0, (GLsizei)w, (GLsizei)h); glMatrixMode(GL_PROJECTION); glLoadIdentity(); gluOrtho2D(0.0, (GLdouble)w, 0.0, (GLdouble)h); } void keyboard(unsigned char key, int x, int y) { switch (key) { case 'c': cohenSutherland(rect, x0, y0, x1, y1); glutPostRedisplay(); break; case 'r': Init(); glutPostRedisplay(); break; case 'x': exit(0); break; default: break; } } int main(int argc, char *argv[]) { glutInit(&argc, argv); glutInitDisplayMode(GLUT_RGB | GLUT_SINGLE); glutInitWindowPosition(100, 100); glutInitWindowSize(640, 480); glutCreateWindow("CohenSutherland algorithm"); Init(); glutDisplayFunc(Display); glutReshapeFunc(Reshape); glutKeyboardFunc(keyboard); glutMainLoop(); return 0; }掌握Cohen-Sutherland裁剪算法的原理及算法,通过示范程序学习,利用OpenGL实现算法。 二、实验内容 (1)根据所给的示范程序,在计算机上编译运行,输出正确结果。 (2)根据给出的示范程序,补全程序其他分区的实现。
时间: 2023-06-14 17:04:15 浏览: 102
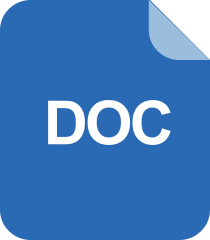
opengl变量设置
(3)在程序中添加键盘事件处理函数,实现按键操作:按下 ‘c’ 键进行裁剪操作,按下 ‘r’ 键进行还原操作,按下 ‘x’ 键退出程序。
实验步骤:
1. 理解Cohen-Sutherland裁剪算法的原理及算法流程;
2. 根据程序框架,补全计算Cohen-Sutherland编码和裁剪算法的函数;
3. 在程序中添加键盘事件处理函数,实现按键操作;
4. 编译运行程序,测试算法的正确性。
补全程序如下:
阅读全文
相关推荐
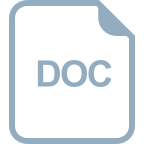
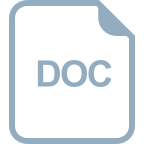
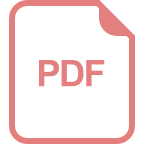
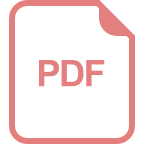


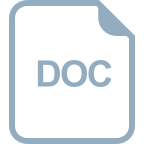
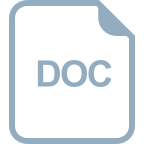
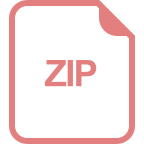
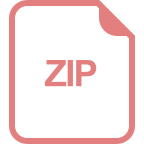
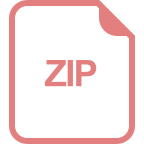
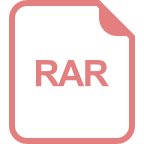
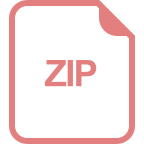
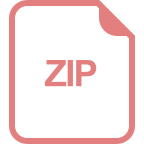
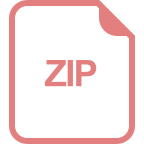
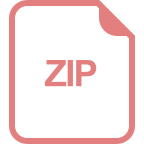
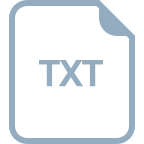
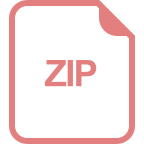