顺序队列出队和入队c语言
时间: 2024-08-13 16:09:41 浏览: 59
在C语言中,顺序队列(也称为线性表)通常使用数组或动态内存分配来实现。出队(删除首元素)和入队(添加新元素到尾部)的操作可以通过下标操作来进行。
**入队操作(Enqueue, 添加元素到队尾):**
1. 首先,检查队列是否已满。如果队列长度小于队列容量,说明还有空间。
2. 将新元素放入数组的最后一个位置,即当前队列长度加一的位置。
3. 如果使用动态内存,可能还需要更新队列的实际长度。
**出队操作(Dequeue, 删除首元素):**
1. 检查队列是否为空。如果队列长度为0,说明没有元素可供删除,这里通常返回一个错误标志或者抛出异常。
2. 记录并移除第一个元素,即从数组的第一个位置开始删除,然后将队列长度减一。
3. 如果动态内存管理,记得释放被删除元素占用的空间。
**C语言代码示例(假设数组表示队列):**
```c
#include <stdio.h>
#define QUEUE_SIZE 10
// 定义队列结构体
typedef struct Queue {
int data[QUEUE_SIZE]; // 数组存储数据
int front; // 前端指针
int rear; // 后端指针
} Queue;
// 出队操作
int dequeue(Queue* queue) {
if (queue->front == queue->rear) { // 空队列
return -1;
}
int removed = queue->data[queue->front];
queue->front = (queue->front + 1) % QUEUE_SIZE; // 循环队列
return removed;
}
// 入队操作
void enqueue(Queue* queue, int value) {
if (queue->rear == (queue->front + QUEUE_SIZE - 1) % QUEUE_SIZE) { // 队列已满
printf("Queue is full.\n");
return;
}
queue->data[queue->rear] = value;
queue->rear = (queue->rear + 1) % QUEUE_SIZE; // 循环队列
}
// 测试示例
int main() {
Queue q;
q.front = q.rear = -1; // 初始化前端和后端指针
enqueue(&q, 1);
enqueue(&q, 2);
enqueue(&q, 3);
printf("dequeue: %d\n", dequeue(&q)); // 输出:dequeue: 1
printf("dequeue: %d\n", dequeue(&q)); // 输出:dequeue: 2
return 0;
}
```
阅读全文
相关推荐
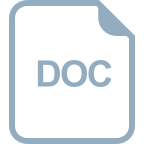
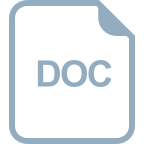
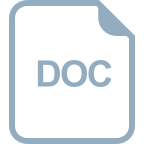


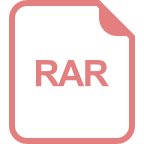
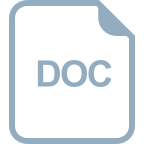











