基于陀螺仪四元数算法c语言
时间: 2023-07-23 14:01:42 浏览: 99
### 回答1:
陀螺仪四元数算法是一种用于姿态估计的算法,通过陀螺仪测量的角速度信息计算出姿态变化,并用四元数表示。以下是基于陀螺仪四元数算法的C语言实现示例:
```c
#include <math.h>
typedef struct {
float w, x, y, z;
} Quaternion;
Quaternion integrateGyro(Quaternion q, float gx, float gy, float gz, float dt) {
Quaternion dq;
float halfT = dt * 0.5f;
float gx2 = gx * halfT;
float gy2 = gy * halfT;
float gz2 = gz * halfT;
dq.w = 1.0f;
dq.x = gx2;
dq.y = gy2;
dq.z = gz2;
q.w += dq.w * q.w - dq.x * q.x - dq.y * q.y - dq.z * q.z;
q.x += dq.w * q.x + dq.x * q.w + dq.y * q.z - dq.z * q.y;
q.y += dq.w * q.y - dq.x * q.z + dq.y * q.w + dq.z * q.x;
q.z += dq.w * q.z + dq.x * q.y - dq.y * q.x + dq.z * q.w;
float norm = sqrtf(q.w * q.w + q.x * q.x + q.y * q.y + q.z * q.z);
q.w /= norm;
q.x /= norm;
q.y /= norm;
q.z /= norm;
return q;
}
int main() {
// 初始化四元数
Quaternion q;
q.w = 1.0f;
q.x = 0.0f;
q.y = 0.0f;
q.z = 0.0f;
// 陀螺仪测量的角速度信息
float gx = 0.1f;
float gy = 0.2f;
float gz = 0.3f;
// 时间间隔
float dt = 0.01f;
// 更新姿态
q = integrateGyro(q, gx, gy, gz, dt);
// 打印更新后的四元数信息
printf("w: %f, x: %f, y: %f, z: %f\n", q.w, q.x, q.y, q.z);
return 0;
}
```
以上是一个简单的基于陀螺仪四元数算法的C语言实现示例。通过调用`integrateGyro`函数,可以根据陀螺仪测量的角速度信息和时间间隔来更新姿态的四元数表示。在主函数中,初始化四元数,给定陀螺仪测量的角速度信息和时间间隔,然后调用`integrateGyro`函数来更新姿态。最后打印更新后的四元数信息。
### 回答2:
陀螺仪四元数算法是一种用于姿态估计的算法,通过利用陀螺仪的测量数据计算出物体的旋转姿态。以下是一个基于陀螺仪四元数算法的C语言实现的示例:
```c
#include <math.h>
typedef struct {
float w, x, y, z;
} Quaternion;
void updateQuaternion(Quaternion* q, float wx, float wy, float wz, float dt) {
Quaternion dq;
dq.w = 0.5 * (-q->x * wx - q->y * wy - q->z * wz) * dt;
dq.x = 0.5 * (q->w * wx + q->y * wz - q->z * wy) * dt;
dq.y = 0.5 * (q->w * wy - q->x * wz + q->z * wx) * dt;
dq.z = 0.5 * (q->w * wz + q->x * wy - q->y * wx) * dt;
q->w += dq.w;
q->x += dq.x;
q->y += dq.y;
q->z += dq.z;
}
void normalizeQuaternion(Quaternion* q) {
float norm = sqrt(q->w * q->w + q->x * q->x + q->y * q->y + q->z * q->z);
q->w /= norm;
q->x /= norm;
q->y /= norm;
q->z /= norm;
}
int main() {
// 初始化四元数为单位四元数
Quaternion quat = {1.0, 0.0, 0.0, 0.0};
// 循环更新四元数
for (int i = 0; i < 100; i++) {
// 模拟陀螺仪测量数据
float wx = 0.1;
float wy = 0.2;
float wz = 0.3;
// 更新四元数
updateQuaternion(&quat, wx, wy, wz, 0.01);
// 归一化四元数
normalizeQuaternion(&quat);
}
return 0;
}
```
上述代码实现了基于陀螺仪测量数据的四元数更新和归一化操作。在循环中,通过调用`updateQuaternion`函数来更新四元数,然后使用`normalizeQuaternion`函数来归一化四元数。这样可以得到物体在空间中的旋转姿态。具体的实现中,还可以根据具体的需求进行其他相关的计算和操作,例如与加速度计数据的融合等。
相关推荐
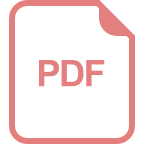











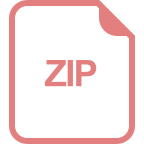
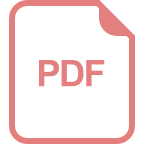