使用C++把检查虚拟机环境的方法全部封装为一个个函数
时间: 2023-12-10 20:06:09 浏览: 24
好的,下面是一个参考实现:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 检查操作系统类型
bool checkOS() {
#ifdef __linux__
cout << "OS: Linux" << endl;
return true;
#elif _WIN32
cout << "OS: Windows" << endl;
return true;
#else
cout << "Unsupported OS." << endl;
return false;
#endif
}
// 检查CPU架构
bool checkCPU() {
#ifdef __x86_64__
cout << "CPU: x86_64" << endl;
return true;
#elif __arm__ || __aarch64__
cout << "CPU: ARM" << endl;
return true;
#else
cout << "Unsupported CPU." << endl;
return false;
#endif
}
// 检查内存大小
bool checkMemory(int minSizeGB) {
long pages = sysconf(_SC_PHYS_PAGES);
long page_size = sysconf(_SC_PAGE_SIZE);
long total_size = pages * page_size;
int total_size_GB = total_size / (1024 * 1024 * 1024);
if (total_size_GB >= minSizeGB) {
cout << "Memory: " << total_size_GB << "GB" << endl;
return true;
} else {
cout << "Insufficient memory." << endl;
return false;
}
}
// 检查硬盘空间
bool checkDiskSpace(int minSpaceGB) {
FILE* f = popen("df -BG / | awk 'NR==2{print $4}'", "r");
if (f == NULL) {
cout << "Failed to execute command." << endl;
return false;
}
char buf[128];
fgets(buf, sizeof(buf), f);
pclose(f);
int freeSpaceGB = stoi(buf);
if (freeSpaceGB >= minSpaceGB) {
cout << "Disk space: " << freeSpaceGB << "GB" << endl;
return true;
} else {
cout << "Insufficient disk space." << endl;
return false;
}
}
// 检查是否安装了指定软件
bool checkSoftware(string softwareName) {
FILE* f = popen(("which " + softwareName).c_str(), "r");
if (f == NULL) {
cout << "Failed to execute command." << endl;
return false;
}
char buf[128];
fgets(buf, sizeof(buf), f);
pclose(f);
string result(buf);
if (result.empty()) {
cout << softwareName << " not found." << endl;
return false;
} else {
cout << softwareName << " found at " << result << endl;
return true;
}
}
int main() {
vector<string> requiredSoftware = {"gcc", "g++", "make", "python3"};
bool osOK = checkOS();
bool cpuOK = checkCPU();
bool memoryOK = checkMemory(8);
bool diskSpaceOK = checkDiskSpace(50);
bool softwareOK = true;
for (string s : requiredSoftware) {
if (!checkSoftware(s)) {
softwareOK = false;
break;
}
}
if (osOK && cpuOK && memoryOK && diskSpaceOK && softwareOK) {
cout << "Virtual machine environment is ready." << endl;
} else {
cout << "Virtual machine environment is not ready." << endl;
}
return 0;
}
```
这里我们使用了 `sysconf` 和 `popen` 来获取系统信息和执行命令。`sysconf` 可以获取系统参数,如内存大小和页面大小,而 `popen` 可以执行命令并获取其输出。在这个实现中,我们使用了 `df` 命令来获取磁盘空间信息,使用了 `which` 命令来检查是否安装了指定的软件。
以上是一个简单的封装检查虚拟机环境的函数的例子,实际应用中还需要根据具体需求进行修改。
相关推荐
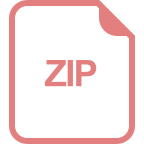
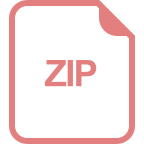













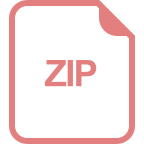
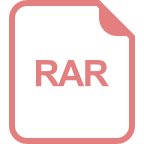